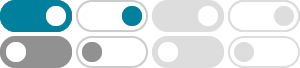
Reading and Writing to text files in Python - GeeksforGeeks
Jan 2, 2025 · There are three ways to read txt file in Python: Reading From a File Using read () read (): Returns the read bytes in form of a string. Reads n bytes, if no n specified, reads the entire file. Reading a Text File Using readline () readline (): Reads a line of the file and returns in form of a string.For specified n, reads at most n bytes.
How to Write to Text File in Python
To write to a text file in Python, you follow these steps: First, open the text file for writing (or append) using the open() function. Second, write to the text file using the write() or writelines() method. Third, close the file using the close() method. The following shows the basic syntax of the open() function: f = open(file, mode)
Python File Write - W3Schools
To write to an existing file, you must add a parameter to the open() function: Open the file "demofile2.txt" and append content to the file: f.write ("Now the file has more content!") Open the file "demofile3.txt" and overwrite the content: f.write ("Woops! I have deleted the content!") Note: the "w" method will overwrite the entire file.
Writing to file in Python - GeeksforGeeks
Dec 19, 2024 · Writing to a file in Python means saving data generated by your program into a file on your system. This article will cover the how to write to files in Python in detail.
python - Print string to text file - Stack Overflow
Jan 26, 2017 · text_file.write("Purchase Amount: %s" % TotalAmount) This is the explicit version (but always remember, the context manager version from above should be preferred): If you're using Python2.6 or higher, it's preferred to use str.format() text_file.write("Purchase Amount: {0}".format(TotalAmount))
How to Use Python to Write a Text File (.txt) - datagy
Jun 20, 2022 · Python provides a number of ways to write text to a file, depending on how many lines you’re writing: These methods allow you to write either a single line at a time or write multiple lines to an opened file.
Writing string to a file on a new line every time - Stack Overflow
Oct 23, 2017 · Use '\\n' to write actual '\n' if needed. You can do this in two ways: or, depending on your Python version (2 or 3): I think the f.write method is better as it can be used in both …
Python Read And Write File: With Examples
Jun 26, 2022 · Files are an essential part of working with computers, thus using Python to write to and read from a file are basic skills that you need to master. In this article, I’ll show you how to do the things you came here for, e.g.: When working with files, there will come that point where you need to know about file modes and permissions.
python - Correct way to write line to file? - Stack Overflow
May 28, 2011 · You should use the print() function which is available since Python 2.6+. For Python 3 you don't need the import, since the print() function is the default. The alternative in Python 3 would be to use: f.write('hi there\n') # python will convert \n to os.linesep. Quoting from Python documentation regarding newlines:
How to Write Data to a File in Python - Tutorial Kart
In Python, you can write data to a file using the open() function in write mode ("w") or append mode ("a"). The write() and writelines() methods allow you to write text or multiple lines efficiently. Let’s explore different ways to write data to a file. 1. Writing a Single Line to a File.