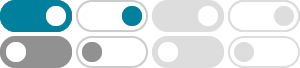
python - How can I add attributes to a module at run time
if you want to set an attribute from the module itself 'at runtime' the correct way is. globals()['name'] = value (Note: this approach only works for globals, not for locals.) if you …
python - How to change a module variable from another module…
Try to import bar.py directly with import bar in __init__.py and conduct your experiment there by setting bar.a = 1. This way, you will actually be modifying bar.__dict__['a'] which is the 'real' …
Python: How to import all methods and attributes from a module ...
Jan 25, 2017 · Is there a way to import all methods and attributes of a module dynamically in Python 2.7? Can you not simply do it with eval? If you have your module object, you can mimic …
How to Import Local Modules with Python - GeeksforGeeks
Jun 28, 2024 · In this article, we will understand how to import local modules with Python. Python Modules: Think of them as individual Python files (e.g., mymodule.py) containing code to …
How to Dynamically Load Modules or Classes in Python
Feb 27, 2020 · In Python we can import modules dynamically by two ways. By using __import__() method: __import__() is a dunder method (methods of class starting and ending with double …
5. The import system — Python 3.13.3 documentation
1 day ago · Python code in one module gains access to the code in another module by the process of importing it. The import statement is the most common way of invoking the import …
A Comprehensive Guide to Importing Modules in Python
Jun 7, 2023 · In this comprehensive guide, we will cover everything you need to know about importing modules in Python, including: Table of Contents. Open Table of Contents. Overview …
importlib — The implementation of import — Python 3.13.3 …
3 days ago · One is to provide the implementation of the import statement (and thus, by extension, the __import__() function) in Python source code. This provides an implementation …
Python Module Attributes: name, doc, file, dict
Python module has its attributes that describes it. Attributes perform some tasks or contain some information about the module. Some of the important attributes are explained below: The …
Customizing Module Behavior in Python: Special Attributes and …
Aug 17, 2024 · These features allow developers to tailor the behavior of their modules, classes, and instances to meet specific requirements. This article will explore some of these special …