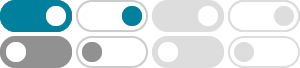
javascript - How to get first character of string? - Stack Overflow
There are many ways to find the string first character in Javascript. I think the easiest way to find is the string.charAt() method. This method takes an index number as a parameter and returns the index value.
Get first letter of each word in a string, in JavaScript
Nov 24, 2020 · Alternative 2: match all the words and use replace() method to replace them with the first letter of each word and ignore the space (the method will not mutate your original string)
javascript - Return First Character of each word in a string - Stack ...
I have a string with Names and I want to convert them to Initials. E.g: John White -> JW John P White -> JPW I am looking for a suggesting on how best to do this. I have researched using split() and
Javascript How to get first three characters of a string
Apr 6, 2018 · I want to get a first three characters of a string. For example: var str = '012123'; console.info(str.substring(0,3)); //012 I want the output of this string '012' but I don't want to use subString or something similar to it because I need to use the …
Finding first alphabetical letter in a string - Javascript
May 13, 2014 · I even tried using a for loop to loop through the string and tried using the functions isLetter() and charAt(). I have a string which is a street address for example: var streetAddr = "45 Church St"; I need a way to loop through the string and find the first alphabetical letter in that string. I need to use that character somewhere else after this.
Keep only first n characters in a string? - Stack Overflow
Oct 10, 2011 · var string = "1234567890" var substr=string.substr(-8); document.write(substr); Output >> 34567890 substr(-8) will keep last 8 chars. var substr=string.substr(8); document.write(substr); Output >> 90 substr(8) will keep last 2 chars. var substr=string.substr(0, 8); document.write(substr); Output >> 12345678 substr(0, 8) will keep first 8 chars
How do I make the first letter of a string uppercase in JavaScript ...
Jun 22, 2009 · p::first-letter { text-transform: uppercase; } Despite being called "::first-letter", it applies to the first character, i.e. in case of string %a, this selector would apply to % and as such a would not be capitalized. In IE9+ or IE5.5+ it's supported in legacy notation with only one colon (:first-letter). ES2015 one-liner
How to use Javascript slice to extract first and last letter of a string?
Nov 13, 2013 · str.split(" "); method splits a String object into an array of strings by separating the string into substrings, where it will find space in string. then str.filter(res=>res.length>0) will filter out string having zero length (for "virat kohali" string you will get empty sub-string) after that using map function you can fetch your first letter
javascript - Getting Name initials using JS - Stack Overflow
Oct 12, 2015 · Then get the first name, and get the first letter: initials = names[0].substring(0, 1).toUpperCase(); If there are more then one name, it takes the first letter of the last name (the one in position names.length - 1 ):
javascript - Get first word of string - Stack Overflow
Sep 1, 2013 · split(" ") will convert your string into an array of words (substrings resulted from the division of the string using space as divider) and then you can get the first word accessing the first array element with [0].