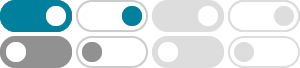
Create and Import modules in Python - GeeksforGeeks
Dec 29, 2019 · In Python, modules allow us to organize code into reusable files, making it easy to import and use functions, classes, and variables from other scripts. Importing a module in Python is similar to using #include in C/C++, providing access to pre-written code and built-in libraries.
class - Employee Classes - Python - Stack Overflow
Import emp.py. from emp import Employee Go ahead and create 3 Employee objects: def main(): emp1 = Employee("name", id, department, title) emp2 = Employee("name", id, department, title) emp3 = Employee("name", id, department, title)
Importing modules inside python class - Stack Overflow
Jul 28, 2011 · If you only want to import them if the class is defined, like if the class is in an conditional block or another class or method, you can do something like this: condition = True if condition: class C(object): os = __import__('os') def __init__(self): print self.os.listdir C.os c = C()
Python class: Employee management system - Stack Overflow
In your case, the class name is Employee within the Employee module, so you would do this: entry = Employee.Employee(id, number, department, job_title) This will create a new Employee object and call its __init__ method with the parameters you passed in.
The Employee Class Program Tutorial | Sophia Learning
Directions: Create our Employee class with the init method parameters and attributes. Also, import the datetime module and create the hiredate attribute. This gives us a starting point with the employee’s details.
GitHub - ANSHEENA-KACHINIKKAD/employee-module-project-: A Python …
To use the Employee class in another part of your code, you can import the employee module. creates an Employee object named "ANU" with a salary of 30000. It then uses the get_name() and get_salary() methods to retrieve and print the employee's information.
How to Import a Class from a File in Python [4 ways] - Python …
Jan 29, 2024 · This article will tell you how to import a class from a file in Python with different ways like user defined class, or how to import all classes from a module, etc
How to Dynamically Load Modules or Classes in Python
Feb 27, 2020 · In Python we can import modules dynamically by two ways By using __import__() method: __import__() is a dunder method (methods of class starting and ending with double underscore also called magic method) and all classes own it.
Let’s get classy: how to create modules and classes with Python
Nov 15, 2018 · A module is a file containing Python definitions and statements. Module is Python code that can be called from other programs for commonly used tasks, without having to type them in each and every program that uses them. For example, when you call “matplotlib.plot”, you are calling a package module. If you didn’t have this module you ...
Create an Employee Class from a List in Python - PyTutorial
Oct 29, 2024 · Learn how to create an Employee class in Python using data from a list. This guide offers examples and explanations for building classes dynamically.