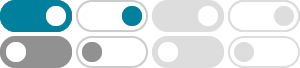
How to 'update' or 'overwrite' a python list - Stack Overflow
An item in a list in Python can be set to a value using the form x[n] = v Where x is the name of the list, n is the index in the array and v is the value you want to set.
Python - Change List Items - W3Schools
To change the value of items within a specific range, define a list with the new values, and refer to the range of index numbers where you want to insert the new values: Change the values "banana" and "cherry" with the values "blackcurrant" and "watermelon":
Update List in Python - GeeksforGeeks
Jan 4, 2025 · The update() method in Python is used to modify dictionaries and sets. For dictionaries, it merges key-value pairs from another dictionary, iterable, or keyword arguments, updating existing keys or adding new ones. For sets, it adds elements from iterables (like lists or tuples), ignoring duplicates
Python: Replacing/Updating Elements in a List (with Examples)
Jun 6, 2023 · If you want to update or replace a single element or a slice of a list by its index, you can use direct assignment with the [] operator. This is usually the simplest and fastest way to modify a list element or slice. Example: print (my_list) # [1, 2, 3, 4, 5] # replace the second element with 10 using indexing . print (my_list)
python - How to modify list entries during for loop ... - Stack Overflow
Sep 8, 2023 · Modifying each element while iterating a list is fine, as long as you do not change add/remove elements to list. You can use list comprehension: l = ['a', ' list', 'of ', ' string '] l = [item.strip() for item in l] or just do the C-style for loop: for …
How to update a list of variables in python? - Stack Overflow
Dec 30, 2016 · What I've done to get around this is just make a function to update/redefine the list that I can call after updating variables. Kind of dirty but it works and is fast. def updateList(): lst = [a,b] This will make the list refresh its contents with the newest variable values, allowing the following: a=1 b=2 list = [a,b] a=2 updateList() print(lst)
Python – Change List Item - GeeksforGeeks
Dec 24, 2024 · Modifying elements in a list is a common task, whether we’re replacing an item at a specific index, updating multiple items at once, or using conditions to modify certain elements. This article explores the different ways to change a list item with practical examples.
How to Update List Element Using Python - Tutorialdeep
Aug 6, 2021 · In this tutorial, learn how to update list element using Python. The short answer is: Use the index position within the square bracket ( [] ) and assign the new element to change any element of the List.
How to Update List Element in Python? - Spark By Examples
May 30, 2024 · To update directly an existing element in a list, with a new value, you can use the assignment (=) operator with the specified index. For example, you can update the element at the index position of 2 in a mylist by assigning the value 35 to mylist[2]. After updating, the list becomes [10, 20, 35, 40, 50]. Yields below output. 3.
Updating Lists in Python - Online Tutorials Library
You can update single or multiple list elements using append, insert, extend, remove, and clear to change the list content by adding, updating, or removing elements from the list object. In this article, we will discuss how we can update an existing element in the list.