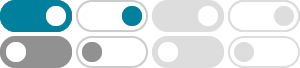
How to pass a list as an input of a function in Python
Feb 1, 2016 · This uses the map function, which takes a list and a function, and returns a new list where that function has been applied individually to each member of the list. In this case, it applies the math.pow(x,2) function to each member of the list, where each number is x.
Passing an array/list into a Python function - Stack Overflow
Dec 17, 2019 · If you want to pass in a List (Array from other languages) you'd do something like this: for x in myList: print x. Then you can call it with this: You need to define named arguments before variable arguments, and variable arguments before keyword arguments. You can also have this: for x in args. print x.
Python - use list as function parameters - Stack Overflow
How can I use a Python list (e.g. params = ['a',3.4,None]) as parameters to a function, e.g.: # do stuff. You can do this using the splat operator: This causes the function to receive each list item as a separate parameter. There's a description here: http://docs.python.org/tutorial/controlflow.html#unpacking-argument-lists.
Pass a List to a Function in Python - GeeksforGeeks
Dec 20, 2024 · When we pass a list as an argument to a function in Python, it allows the function to access, process, and modify the elements of the list. In this article, we will see How to pass a list as an argument in Python, along with different use cases.
Get a list as input from user in Python - GeeksforGeeks
Dec 5, 2024 · In this article, we will see how to take a list as input from the user using Python. Get list as input Using split() Method The input() function can be combined with split() to accept multiple elements in a single line and store them in a list.
Python Passing a List as an Argument - W3Schools
Passing a List as an Argument. You can send any data types of argument to a function (string, number, list, dictionary etc.), and it will be treated as the same data type inside the function. E.g. if you send a List as an argument, it will still be a List when it reaches the function:
Python – Passing list as an argumnet - GeeksforGeeks
Dec 26, 2024 · When we pass a list as an argument to a function in Python, it allows the function to access, process, and modify the elements of the list. In this article, we will see How to pass a list as an argument in Python, along with different use cases. We can directly pass a list as a function argument.
5 Best Ways to Pass a Python List to Function Arguments
Feb 20, 2024 · This article deals with converting a Python list into function arguments effectively. Consider a function def add(a, b, c): that expects three arguments. The input might be a list [1, 2, 3] , and the desired output is the result of add(1, 2, 3) .
How to Pass List to Function in Python - Delft Stack
Feb 2, 2024 · In Python, you can pass the elements of a list as multiple arguments to a function using the asterisk (*) operator. This technique is known as unpacking, and it allows you to pass each element of the list as an individual argument to the function.
How to Pass a List to a Function in Python
Nov 19, 2023 · In this code, we’re passing a list [‘Alice’, ‘Bob’, ‘Charlie’] to the greetings () function. The function will then print out “Hello, Alice”, “Hello, Bob”, and “Hello, Charlie”. This is a simple demonstration of how you can pass multiple values to a function in Python using a list.