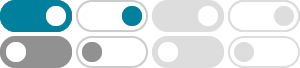
Sorting an array in Bash using Bubble sort - GeeksforGeeks
Nov 25, 2021 · Given an array arr sort the array in ascending order using bash scripting. Examples: Approach : For sorting the array bubble sort is the simplest technique. Bubble sort works by swapping the adjacent elements if they are in the wrong order. Example: and so on...
Bubble sort in Linux/Unix shell scripting - Stack Overflow
Feb 8, 2013 · fix that, your program will sort. read n; #get the size of array from user. flag=0; for ((j = 0; j < $n-1-$i; j++ )) do. if [[ ${arr[$j]} -gt ${arr[$j+1]} ]] then. temp=${arr[$j]}; arr[$j]=${arr[$j+1]}; arr[$j+1]=$temp; flag=1; fi. done. if [[ $flag -eq 0 ]]; then. break; …
How to write Bubble sort in Bash - The Geek Diary
Here is a simple bash script for bubble sort algorithm. read nos[$i] echo ${nos[$i]} for (( j = $i; j < $n; j++ )) do. if [ ${nos[$i]} -gt ${nos[$j]} ]; then. t=${nos[$i]} nos[$i]=${nos[$j]} nos[$j]=$t. fi. done. echo ${nos[$i]} Sample Output from the above script:
Bubble Sort Example Using Bash Script – BashScript.net
Dec 12, 2024 · Learn how to implement a Bubble Sort algorithm using Bash scripting. Bubble Sort is a simple sorting technique that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
linux - Bubble sort bash script - Stack Overflow
I am trying to sort a list of names and their scores in dictionary order in bash using bubble sort. The arrays are. The names are stored in an array names, and the scores are in an array scores. This is my code and its giving me an error on line 30 saying "to many arguments" and I cannot seem to find why. echo "$1 must be a file" exit 1.
bubble sort on command line arguments in bash - Stack Overflow
Oct 5, 2017 · I need to create a bubble sort to take in command line arguments in bash (ints) and print out them in ascending order. I believe I implemented the algorithm correctly, but I'm having trouble getting the array of the command like arguments working.
Shell Script of Bubble sort · GitHub
echo "Enter number of elements" read k: echo "enter array elements" for ((i=0; i<k; i++)) do: read a[$i] done: for ((i=0; i<k; i++)) do: for((j=$i; j<k; j++)) do: if [ ${a[$i]} -gt ${a[$j]} ] then: …
Write a shell script to sort a set of integer numbers using bubble sort …
Write a shell script to sort a set of integer numbers using bubble sort an anonymous user · December 14, 2022 Bash Bash 5.1.16 Run Fork Copy link Download Share on Facebook Share on Twitter Share on Reddit Embed on website arr=(11 44 55 22 33) echo "Array in original order" echo ${arr[*]} for((i=0; i<5; i++)) do for((j=0; j<5-i-1;j++)) do if ...
Bubble Sort Bash Script - Ask Ubuntu
Jun 22, 2017 · Script: #!/bin/bash bubbleSort() { for ((i=0;i<=${#nums[@]};i++)) do for ((j=0;j<=${#nums[@]};j++)) do if [ ${nums[$i]} -gt ${nums[$j]} ] then t=${nums[$i]} nums[$i]=${nums[$j]} nums[$j]=$t fi done done } declare -a nums=(89 62 11 75 8 33 95 4) echo ${nums[*]} #prints out all elems bubbleSort echo ${nums[*]} #prints out all elems
How to implement bubble sort in a shell script? | LabEx
To implement bubble sort in a shell script, we can follow these steps: Define a function to perform the bubble sort. Iterate through the array and compare adjacent elements. Swap the elements if they are in the wrong order. Repeat the process until the entire array is sorted. Here's an example implementation in a shell script: