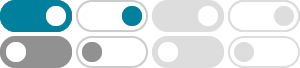
Remove all consecutive duplicates from the string
Aug 22, 2023 · Given a string S, The task is to remove all the consecutive duplicate characters of the string and return the resultant string. Note: that this problem is different from Recursively remove all adjacent duplicates. Here we keep one character and remove all subsequent same characters. Examples: Approach: 1) Input String S.
Removing duplicates from a String in Java - Stack Overflow
Java 8 has a new String.chars () method which returns a stream of characters in the String. You can use stream operations to filter out the duplicate characters like so: but you cant use it if the input has the same elements, or if its empty! Code to remove the duplicate characters in a string without using any additional buffer.
How to Remove Duplicates from a String in Java? - GeeksforGeeks
Feb 12, 2024 · Working with strings is a typical activity in Java programming, and sometimes we need to remove duplicate characters from a string. Using hashing is one effective way to do this. By assigning a unique hash code to each element, …
Java Program To Remove Duplicates From A Given String
Oct 13, 2023 · Write a java program for a given string S, the task is to remove all the duplicates in the given string. Below are the different methods to remove duplicates in a string. Note: The order of remaining characters in the output should be the same as in the original string. Example: Input: Str = geeksforgeeks Output: geksfor
How to efficiently remove consecutive same characters in a string
Jul 18, 2021 · Here is a way to solve this: StringBuilder answer = new StringBuilder(""); int N = input.length(); int i = 0; while (i < N) char c = input.charAt(i); answer.append( c ); while (i<N && input.charAt(i)==c) ++i; return answer.toString();
Java program to remove consecutive duplicate characters from string
Jan 19, 2024 · public static String removeConsecativeDuplicat(String input) { StringBuilder result = new StringBuilder(); for (int i = 2; i < input.length(); i++) { if (input.charAt(i) != input.charAt(i - 1) & input.charAt(i - 1) != input.charAt(i - 2)) { result.append(input.charAt(i)); return result.toString();
Java program to remove duplicate characters from a string
We can remove the duplicate characters from a string by using the simple for loop, sorting, hashing, and IndexOf () method. So, there can be more than one way for removing duplicates. By using the simple for loop. By using the sorting algorithm. By using the hashing. By using the indexOf () method.
5 ways to remove duplicate characters from java string - codippa
Mar 28, 2020 · Learn different methods to remove repeated characters from a string in java with example programs, explanation and output.
Removing Repeated Characters from a String - Baeldung
Jan 8, 2024 · In this article, we covered a few ways to remove repeated characters from a string in Java. We also looked at the time and space complexity of each of these methods.
Remove duplicates from a string - GeeksforGeeks
Sep 11, 2024 · Given a string s which may contain lowercase and uppercase characters. The task is to remove all duplicate characters from the string and find the resultant string. Note: The order of remaining characters in the output should be the same as in the original string. Example: