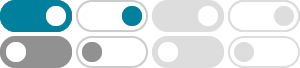
C program to Find the Largest Number Among Three Numbers
Mar 20, 2025 · The basic method to find largest number among three numbers is using if statement to compare the numbers with each other in pairs. Lets consider three numbers a, b, and c: Check if a is greater than to b and a is also greater than to c .
Write a C Program to find largest number using pointers
Nov 29, 2016 · Write a C Program to find largest number using pointers. Here’s simple Program to find largest number using pointers in C Programming Language. What are Pointers? A pointer is a variable whose value is the address of another …
C Program to Find the Largest of three numbers using Pointers
Feb 25, 2019 · In this tutorial, we will write a C program to find the largest of three input numbers using pointers. In the following program we have three integers num1, num2 & num3. We have assigned the addresses of these three numbers to three pointers p1, p2 & p3 respectively.
C Program to Find Largest Element in an Array using Pointers
Jun 8, 2023 · In this article, we will learn how to find the largest element in the array using a C program. The simplest method to find the largest element in the array is by iterating the array and comparing each element with the assumed maximum and updating it when the element is greater.
C Program to Find the Largest of Two Numbers using a Pointer
Write a C program to find the largest of two numbers using a pointer. It allows the user to enter two integer values, and then we assign them to two pointer variables of int type. In this example, we used the else if statement to find the largest of two pointer numbers.
C Program to Find Largest of Three Numbers Using Pointer
This C program finds largest of three numbers using pointer. pa = & a; . pb = & b; . pc = & c; if(* pa > * pb && * pa > * pc) { printf("Largest is: %d", * pa); } else if(* pb > * pc && * pb > * pc) { printf("Largest is : %d", * pb); } else { printf("Largest = %d", * pc); } return 0; } Note: ↲ indicates enter is pressed.
C Program to Find Largest Number Using Dynamic Memory Allocation
In this C programming example, you will learn to find the largest number entered by the user in a dynamically allocated memory.
Find maximum of three number in C without using conditional …
Aug 16, 2011 · #Python program to find the largest among three integer numbers using ternary operators: x=int(input("enter 1st number: ")) y=int(input("enter second number: ")) z=int(input("enter third number: ")) large=(x if x>z else z ) if x>y else (y if y>z else z) print(large ,"is the largest number.")
C Program to Find the Largest Number Among Three Numbers
In this example, you will learn to find the largest number among the three numbers entered by the user in C programming. Learn to code solving problems and writing code with our hands-on C Programming course.
C Program to Find Largest Element in an Array
In this C programming example, you will learn to display the largest element entered by the user in an array.