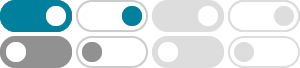
Stack in Python - GeeksforGeeks
Jun 20, 2024 · A stack is a linear data structure that stores items in a Last-In/First-Out (LIFO) or First-In/Last-Out (FILO) manner. In stack, a new element is added at one end and an element is removed from that end only. The insert and delete operations are often called push and pop.
Implementation of Stack in Python using List - GeeksforGeeks
4 days ago · push(self, st, data): Adds an element to the top of the stack (list) using append(). pop(self, st): Removes the top element from the stack using pop(), and prints the removed element. It also checks if the stack is empty before performing the operation.
Stack Data Structure and Implementation in Python, Java and …
There are some basic operations that allow us to perform different actions on a stack. Push: Add an element to the top of a stack; Pop: Remove an element from the top of a stack; IsEmpty: Check if the stack is empty; IsFull: Check if the stack is full; Peek: Get the value of the top element without removing it
Implement Stack using Array - GeeksforGeeks
Mar 21, 2025 · Implement push (add to end), pop (remove from end), and peek (check end) operations, handling cases for an empty or full stack. Step-by-step approach: Initialize an array to represent the stack. Use the end of the array to represent the top of the stack.
Push and Pop Operation in Stack: Algorithm and Program
Apr 5, 2021 · PUSH operation of stack is used to add an item to a stack at top and POP operation is performed on the stack to remove items from the stack.
Python Stack Data Structure: When and Why to Use it
1 day ago · A stack in Python is a type of collection used to organize and manage data in a specific order. Elements in a stack are added and removed from only one end, called the top. ... The two key operations that make a stack work are: Push: This operation adds a new item to the top of the stack. ... DFS algorithms use stacks to remember which node to ...
Stack Push Operation - Python Examples
This Python program defines a stack with methods for pushing elements onto the stack and traversing the stack. The push method creates a new node, sets its next reference to the current top of the stack, and updates the top reference to the new node.
Implement a Stack Data Structure in Python - Push, Pop, Peek
Aug 4, 2023 · In this comprehensive guide, you will learn how to implement a stack data structure in Python using arrays, linked lists, or Python’s built-in list type. We will cover the key stack operations like push, pop, and peek with example code snippets. You will also learn various applications of stacks that demonstrate their utility.
A Complete Guide to Stacks in Python
Apr 9, 2025 · A tutorial on stacks in Python. | Video: codebasics. More on Python Python Regular Expression re.match() and re.sub() Explained. Disadvantages of Stack in Python. Limited flexibility: Since stacks operate in LIFO order, accessing elements other than the top element is inefficient.; Memory usage: If not managed properly, stacks can cause excessive memory consumption, leading to stack overflow ...
Python OOP: Stack class with push, pop, and display methods
Mar 29, 2025 · We push and pop several items onto the stack using the "push ()" and "pop ()" methods. We then display the stack elements using the "display ()" method. Write a Python class for a Stack that includes methods for push, pop, and a method to display all elements in the stack.
- Some results have been removed