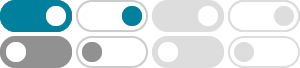
Split a number from a string in JavaScript - Stack Overflow
Mar 16, 2017 · Then you need to use a regex-based split with a positive lookahead with a digit matching pattern. Second solution: /* letr[0] will be foofo. Now both are separated, and you can make any string as you like. */ You want a very basic regular expression, (\d+). This will match only digits. Perfect one-liner. This should be the accepted answer.
Extract a Number from a String using JavaScript
Jan 9, 2025 · Using Array.prototype.filter with split, you can extract numbers from a string by splitting the string into an array of characters, filtering out non-numeric characters, and joining the remaining characters back into a string.
Separate characters and numbers from a string - Stack Overflow
Jun 17, 2016 · A shorter solution if the string always starts with letters and ends with numbers as you say: var str = 'aaaaa12111'; var chars = str.slice(0, str.search(/\d/)); var numbs = str.replace(chars, ''); console.log(chars, numbs);
JavaScript String split() Method - W3Schools
The split() method splits a string into an array of substrings. The split() method returns the new array. The split() method does not change the original string. If (" ") is used as separator, the string is split between words.
Separate characters and numbers from a string in JavaScript
Learn how to separate characters, special characters, and numbers from a string using match(), split() and replace() method in JavaScript.
Split a number into individual digits using JavaScript
Jun 27, 2024 · There are several approaches to splitting a number into individual digits using JavaScript which are as follows: In this approach number.toString () converts the number to a string, split (”) splits the string into an array of characters, and map (Number) converts each character back to a number.
JavaScript String slice() Method - W3Schools
The slice() method returns the extracted part in a new string. The slice() method does not change the original string. The start and end parameters specifies the part of the string to extract.
String.prototype.split() - JavaScript | MDN - MDN Web Docs
Apr 3, 2025 · The split() method of String values takes a pattern and divides this string into an ordered list of substrings by searching for the pattern, puts these substrings into an array, and returns the array.
Extract a Number from a String Using JavaScript - Online …
In JavaScript, there are multiple ways to extract a number from a string. One way is to use the match() method and a regular expression to search for all numeric digits in the string. Another way is to use the replace() method and a regular expression to remove all nonnumeric characters from the string, leaving only the numbers.
The Ultimate Guide to JavaScript‘s Powerful split() Method
Jan 1, 2025 · In this ultimate guide, you‘ll learn: So let‘s dive in and uncover the full power of split ()! The basic syntax for split () is: It takes a string, splits it based on the separator param, and returns a new array. The separator can be any string or regular expression. Split () will look for that pattern in the string and break it up around matches.
- Some results have been removed