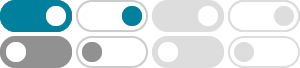
Recursively Reverse the Elements in an Array - Stack Overflow
Jan 18, 2015 · I want to write a recursive function in javascript that returns an array with its elements reversed. This code generates the following error: var reversed = []; function reverser (toBeReversed){ if (toBeReversed.length == 1) reversed.push(toBeReversed[0]); else { reversed.push(toBeReversed.lastOfIndex(0)); //error on this line.
What is the most efficient way to reverse an array in JavaScript?
// array-reverse-polyfill.js v1 Array.prototype.reverse = Array.prototype.reverse || function() { return this.sort(function() { return -1; }); }; If you are in a modern browser you use the native reverse function.
JavaScript – Sort an Array in Reverse Order in JS
Nov 15, 2024 · JS array.sort () Method sorts the array in ascending order. We can make it descending or reverse order using array.reverse () method. Below are the following ways to sort an array in reverse order: 1. Using array.sort () with array.reverse () First, sort the given array of strings using JS array.sort () method.
Recursive Array Reversal Javascript - Stack Overflow
Nov 12, 2014 · return arr.length < 2 ? arr : [arr.pop()].concat(reverse(arr)); Here's a runnable example with a working function. FYI there is also a built-in function that will do this for you.
Reverse an Array in JavaScript - GeeksforGeeks
Jan 20, 2025 · You can use recursion by removing the first element of the array, reversing the rest of the array, and then pushing the removed element to the end of the reversed array. The recursive function removes the last element of the array using pop () and appends it …
Sorting Algorithms in JavaScript - GeeksforGeeks
Feb 12, 2025 · Merge Sort is a divide-and-conquer algorithm. It divides the array into two halves, recursively sorts them, and merges the sorted halves. 3, 9, 10, 27, 38, 43, 82. Quick Sort is another divide-and-conquer algorithm.
Reversing an Array Using Direct Recursion in JavaScript - LinkedIn
Jan 6, 2025 · Here, we'll explore how to reverse an array using direct recursion in JavaScript. Recursion is a method of solving problems where a function calls itself as a subroutine. This approach is...
How to Reverse an Array in JavaScript | TheDevSpace Community
This tutorial discusses four different ways you can reverse an array in JavaScript, including the reverse() method, for loops, recursions, and the map() method.
JavaScript program to reverse an array using recursion
Aug 16, 2022 · let arr = [1, 2, 3, 4, 5]; let reverseArray = (arr, left, right) => { if (left >= right) { ... Tagged with javascript, beginners, programming, tutorial.
JavaScript Reverse Array – A Comprehensive Tutorial
Aug 30, 2024 · In this comprehensive tutorial, we‘ll explore 5 methods for reversing arrays in JavaScript: We‘ll analyze the performance, use cases, and limitations of each approach. By the end, you‘ll thoroughly understand the various methods available for array reversal in JavaScript. Why Learn Array Reversal?
- Some results have been removed