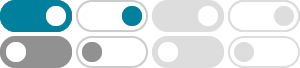
python - How can I use `return` to get back multiple values from a loop …
Jul 4, 2020 · Using a return inside of a loop will break it and exit the function even if the iteration is still not finished. For example: # Here there will be only one iteration. # For number == 1 => 1 % 2 = 1. # So, break the loop and return the number. for number in range(1, 10): if …
python - return statement in for loops - Stack Overflow
If you want the function to run for however many times you tell it to, you have to put the return AFTER the for-loop, not inside it. That way, the function will return after the control gets off the loop
python - How do I get ("return") a result (output) from a function…
Effectively, there are two ways: directly and indirectly. The direct way is to return a value from the function, as you tried, and let the calling code use that value. This is normally what you want. The natural, simple, direct, explicit way to get information back from a function is to return it.
Python return statement - GeeksforGeeks
Dec 10, 2024 · Python allows you to return multiple values from a function by returning them as a tuple: Example: In this example, the fun () function returns two values: name and age. The caller unpacks these values into separate variables. We can also return more complex data structures such as lists or dictionaries from a function:
Python For Loops - W3Schools
With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc. Print each fruit in a fruit list: The for loop does not require an indexing variable to set beforehand. Even strings are iterable objects, they contain a sequence of characters: Loop through the letters in the word "banana":
Python Language Tutorial => Return statement inside loop in a function
print ('Got value {}'.format(value)) if value == 1: # Returns from function as soon as value is 1. print (">>>> Got 1") return. print ("Still looping") return "Couldn't find 1" Got any Python …
The Python return Statement: Usage and Best Practices
In this tutorial, you’ll learn that: You use return to send objects from your functions back to the caller code. You can use return to return one single value or multiple values separated by commas. You should try to keep your code readable and …
returning values in for loop - Python Forum
Dec 17, 2020 · Use yield instead of return. This turns example () into a generator, like range (). Then you could do this: If you really just want to return the list. Just return the list. A function can only return one object. If you want multiple values, you have to return it in a collection object of some sort (list, tuple, set, dict, etc.)
Returning a function from a function - Python - GeeksforGeeks
Mar 19, 2025 · Returning functions from functions is a versatile and powerful feature in Python. It can be used in various scenarios, such as: Callbacks: Functions can be returned to be called later in response to some event. Closures: Functions can capture variables from the enclosing scope, enabling encapsulation and maintaining state across function calls.
function - How to return value from For loop in python? - Stack Overflow
Jan 29, 2016 · def getvalues(): values = [] dictt = getvalues() for key , value in getvalues.iteritems(): values.append((key, getvalues[key])) return values