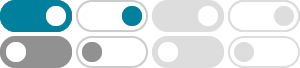
Reversing a String with Recursion in Java - Stack Overflow
Here is some Java code to reverse a string recursively. Could someone provide an explanation of how it works? public static String reverse(String str) { if ((null == str) || (str.length() &...
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · Given a string, the task is to print the given string in reverse order using recursion. Examples: Input: s = “Geeks for Geeks” Output: “ skeeG rof skeeG “ Explanation: After reversing the input string we get “ skeeG rof skeeG “. Input: s = “Reverse a string Using Recursion” Output: “ noisruceR gnisU gnirts a esreveR “
Whats the best way to recursively reverse a string in Java?
May 14, 2009 · I set out to recursively reverse a string. Here's what I came up with: //A method to reverse a string using recursion public String reverseString(String s){ char c = s.charAt(s.length()-1); if(s.length() == 1) return Character.toString(c); return c …
Java Program to Reverse a String Using Recursion
Mar 11, 2021 · In this program, we will see how to reverse a string using recursion with a user-defined string. Here, we will ask the user to enter the string and then we will reverse that string by calling a function recursively and finally will print the reversed string. Declare a string. Ask the user to initialize the string.
Reverse a String – Complete Tutorial - GeeksforGeeks
Jan 29, 2025 · Inside the recursive function, Swap the first and last element. Recursively call the function with the remaining substring. The idea is to use stack for reversing a string because Stack follows Last In First Out (LIFO) principle. This means the last character you add is the first one you’ll take out.
Reverse a String Using Recursion in Java - Tpoint Tech
Mar 26, 2025 · In this section, we will learn how to reverse a string using recursion in Java. First, remove the first character from the string and append that character at the end of the string. Repeat the above step until the input string becomes empty. Suppose, the input string JAVATPOINT is to be reversed.
how java uses recursion to reverse a string - Stack Overflow
Jul 18, 2014 · Here is a recursive method: //base case to handle one char string and empty string. if (str.length() < 2) { return str; System.out.println(str.substring(1) + " "+ str.charAt(0)); return reverseRecursively(str.substring(1)) + str.charAt(0); Obviously it is supposed to reverse a String. The output I get from this method is this: It works fine.
Java Program to Reverse a String using Recursion
Sep 8, 2017 · We will see two programs to reverse a string. First program reverses the given string using recursion and the second program reads the string entered by user and then reverses it. Output: str = scanner.nextLine(); .
How to Reverse a String in Java using Recursion - Guru99
Dec 30, 2023 · In this example program, we will reverse a string entered by a user. We will create a function to reverse a string. Later we will call it recursively until all characters are reversed.
Reverse A String Using Recursion - Java Code Geeks
Jun 30, 2020 · In this article, You’re going to learn how to reverse a string using recursion approach. The first program is to reverse a string and the second program will read the input from the user. In the previous articles, I have shown already how to reverse a string without using any built-in function and also how to reverse the words in a string .
- Some results have been removed