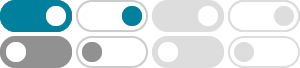
Fibonacci Series without Recursion in Python - Sanfoundry
This is a Python Program to find the fibonacci series without using recursion. The program takes the first two numbers of the series along with the number of terms needed and prints the fibonacci series. 1. Take the first two numbers of the series and the number of terms to be printed from the user. 2. Print the first two numbers. 3.
5 Best Ways to Compute the Fibonacci Series without Recursion in Python
Mar 7, 2024 · A generator function in Python uses the keyword yield instead of return to provide a result without stopping its execution. This is particularly useful for generating sequences as it allows for iteration over each element without storing the entire sequence in memory.
Find Fibonacci Series Without Using Recursion in Python
Mar 12, 2021 · Learn how to find the Fibonacci series without using recursion in Python with this simple guide and example code.
Print the Fibonacci sequence – Python | GeeksforGeeks
Mar 22, 2025 · Using Recursion . This approach uses recursion to calculate the Fibonacci sequence. The base cases handle inputs of 0 and 1 (returning 0 and 1 respectively). For values greater than 1, it recursively calls the function to sum the previous two Fibonacci numbers.
Fibonacci Series Program in Python - Python Guides
Aug 27, 2024 · In this Python tutorial, we covered several methods to generate the Fibonacci series in Python, including using for loops, while loops, functions, recursion, and dynamic programming. We also demonstrated how to generate the Fibonacci series up to a specific range without using recursion.
Python Program to Find the Fibonacci Series Without using Recursion
Nov 20, 2024 · This Python program generates the Fibonacci series without using recursion. It calculates and displays the first n Fibonacci numbers using iteration. The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones.
python - Efficient calculation of Fibonacci series - Stack Overflow
Aug 11, 2013 · This formula can calculate the nth number of the fibonacci sequence easily in O(1) time. Here is my Java code translated to Python: def fibonacci(n): five_sqrt = 5 ** 0.5 return int(round((((1 + five_sqrt)/2) ** n)/five_sqrt)) for i in range(1, 21): print(fibonacci(i)) Output:
Fibonacci Sequence: Iterative Solution in Python
In Python, we can solve the Fibonacci sequence in both recursive as well as iterative ways, but the iterative way is the best and easiest way to do it. The source code of the Python Program to find the Fibonacci series without using recursion is given below. c=a+b. a,b = …
Designing Code for Fibonacci Sequence without Recursion
First few Fibonacci numbers are 0 1 1 2 3 5 8 …..etc. Let us now write code to display this sequence without recursion. Because recursion is simple, i.e. just use the concept, Fib (i) = Fib (i-1) + Fib (i-2) However, because of the repeated calculations in recursion, large numbers take a long time. So, without recursion, let’s do it. Approach:
python - An iterative algorithm for Fibonacci numbers - Stack Overflow
Feb 24, 2013 · Non recursive Fibonacci sequence in python. def fibs(n): f = [] a = 0 b = 1 if n == 0 or n == 1: print n else: f.append(a) f.append(b) while len(f) != n: temp = a + b f.append(temp) a = b b = temp print f fibs(10)
- Some results have been removed