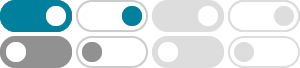
Bubble Sort - Python - GeeksforGeeks
Feb 21, 2025 · Bubble Sort algorithm, sorts an array by repeatedly comparing adjacent elements and swapping them if they are in the wrong order. The algorithm iterates through the array multiple times, with each pass pushing the largest unsorted element to its …
Bubble Sort Algorithm: Pseudocode and Explanation
Here’s the pseudocode for the iterative approach to bubble sort, using Python-like syntax: # Get the length of the array . n = len (array) # Outer loop: Iterate through the array # After each pass, the largest unsorted element "bubbles" to its correct position at the end for i in range (n):
Pseudocode and Flowchart for Bubble Sort - ATechDaily
Mar 7, 2021 · In this article, we will understand the Pseudocode [Algorithm for Bubble Sort, Pseudocode for Bubble Sort, Flowchart for Bubble Sort, Simple Bubble Sort Algorithm Explanation]
Bubble Sort in Computer Science - IGCSE Revision Notes - Save …
Apr 8, 2025 · A bubble sort is a simple sorting algorithm that starts at the beginning of a dataset and checks values in 'pairs' and swaps them if they are not in the correct order. One full run of comparisons from beginning to end is called a 'pass', a bubble sort may require multiple 'passes' to sort the dataset. The algorithm is finished when there are no ...
Bubble Sort (With Code in Python/C++/Java/C) - Programiz
The bubble sort algorithm compares two adjacent elements and swaps them if they are not in the intended order. In this tutorial, we will learn about the working of the bubble sort algorithm along with its implementations in Python, Java and C/C++.
python - Bubble sort implementation from Pseudocode - Stack Overflow
Here is the pseudocode I have. i <- n. last <- 1. while i > last do. for j <- 1 to i-1 do. if t[j] > t[j+1] do. t[j] <-> t[j+1] {switch values} last <- j. end if. end for. i <- last. last <- 1. end while. I just need to state why this is an improvement on the standard bubble sort and do …
Bubble Sort: A Simple and Efficient Sorting Algorithm for Python
Mar 17, 2023 · Here is a simple implementation of the bubble sort algorithm in Python: This follows the bubble sort pseudocode directly. We iterate through the list while swapping any adjacent elements that are out of order, repeating this process until the list is fully sorted.
Bubble Sort — How It Works, Psuedocode and C++ & Python
Nov 20, 2021 · In this article we’ll look into how Bubble Sort works by looking into the psudeocode and actual implementation of it. Suppose we need to sort a sequence of elements into non-decreasing order...
Bubble Sort in Python
Apr 18, 2022 · For example, at the end of the first step, the left-most element is sorted, at the end of the second step the two most-left elements in the array are sorted, etc. Finally, after n-1 steps, all the elements have been sorted. The pseudocode of the Bubble Sort algorithm is the following: 1. procedure bubble_sort(data_list): 2. n = length of list 3.
Bubble Sort - Fully Understood (Explained with Pseudocode)
Sep 9, 2019 · Bubble sort is a sorting algorithm that finds max. element in each cycle and puts it in appropriate position in list by swapping adjacent elements.
- Some results have been removed