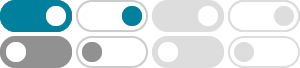
python - Reverse list using for loop - Stack Overflow
Feb 10, 2018 · List can be reversed using for loop as follows: >>> def reverse_list(nums): ... # Traverse [n-1, -1) , in the opposite direction. ... for i in range(len(nums)-1, -1, -1): ... yield nums[i] ... >>> >>> print list(reverse_list([1,2,3,4,5,6,7])) [7, 6, 5, 4, 3, 2, 1] >>> Checkout this link on Python List Reversal for more details
loops - Traverse a list in reverse order in Python - Stack Overflow
Feb 10, 2009 · How do I traverse a list in reverse order in Python? So I can start from collection[len(collection)-1] and end in collection[0]. I also want to be able to access the loop index.
Print a list in reverse order with range ()? - Stack Overflow
Sep 2, 2011 · Use reversed() function (efficient since range implements __reversed__): It's much more meaningful. Update: list cast. If you want it to be a list (as @btk pointed out): Update: range -only solution. If you want to use only range to achieve the same result, you can use all its parameters. range(start, stop, step)
Reversing a List in Python - GeeksforGeeks
Oct 15, 2024 · Python provides several ways to iterate over list. The simplest and the most common way to iterate over a list is to use a for loop. This method allows us to access each element in the list directly. Example: Print all elements in the list one by one using for loop. [GFGTABS] Python a = [1, 3, 5, 7,
Python - Print list in reverse order - Python Examples
In Python, you can print the elements in a list in reverse order using a For loop with reversed list, or a While loop with a counter for index that traverses from ending of the list to starting of the list.
How to Reverse a List in Python [Using While and For Loop] - Python …
Apr 19, 2024 · In this Python tutorial, I will explain how to reverse a list in Python using while loop and for loop. The reverse of data in the list is used in data processing or any other fields where the order of operations needs to be reversed in functional programming contexts.
Python : Different ways to Iterate over a List in Reverse Order
let us use list comprehension to print list in reverse order. Below is the implementation: # Given list givenlist = ['hello', 'world', 'this', 'is', 'python'] # Iterate over the list using List Comprehension and [::-1] [print(element) for element in reversed(givenlist)]
How to Loop Through a List Backwards in Python - Tutorial Kart
To loop through a list backwards in Python, you can use methods like reversed(), slicing ([::-1]), the range() function, or the iter() function with reversed(). 1. Using the reversed() Function. The built-in reversed() function returns an iterator that allows iterating over …
How to Reverse a List in Python Using for loop
Steps to Reverse a List Using for-loop. Take your list (my_list = [1,2,3,4,5]). Create an empty list to store elements in reversed order (r_list = []). Using for loop iterate in the list in reversed order (for i in range(len(my_list)-1,-1,-1)). Inside the loop append i th element in the empty list (r_list.append(my_list[i])). print reversed ...
Iterate over a List or String in Reverse order in Python
Apr 9, 2024 · To iterate over a list in reverse order with index: Use the enumerate() function to get access to the index. Convert the enumerate object to a list and reverse the list. Use a for loop to iterate over the list in reverse order. We used the enumerate function …