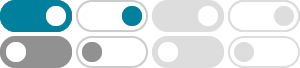
Java Program to Print Triangle of Alphabets in Reverse Pattern
Write a Java program to print triangle of alphabets in reverse pattern using for loop. private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); . System.out.print("Enter Triangle of Alphabets in Reverse Rows = "); int rows = sc.nextInt(); System.out.println("Printing Triangle of Alphabets in Reverse Order");
Java Pattern Programs – Learn How to Print Pattern in Java
Apr 8, 2025 · Pyramid patterns is a classic logical programming exercise where a triangular looking pattern is printed by treating the output screen as a matrix and printing a given character. In this article, we will explore how to print various alphabet pyramid patterns using C program. Half Pyramid PatternHalf
beginner java - printing a right triangle backwards
"Write a program that will draw a right triangle of 100 lines in the following shape: The first line, print 100 ' ', the second line, 99 ' ’... the last line, only one '*'. Using for loop for this problem. Name the program as PrintTriangle.java" *****
Java Program to Print Right Triangle of Alphabets in Reverse
This Java pattern program prints the right angled triangle of alphabets in reverse order or descending order using while loop.
Printing Reverse Triangle with Numbers - Java - Stack Overflow
Sep 24, 2014 · You just need to reverse the order of your loops and decrement x instead of incrementing it. I change the code a bit though : int level = 3; for(int r=level ; r>0 ; r--) { for(int c=r ; c>0 ; c--) for (int x=0 ; x<c ; x++) System.out.print("*"); System.out.println(); }
Top 10 Character pattern programs in java - Javacodepoint
In this article, you will see the Top 10 Character/Alphabet pattern programs logic in java. We recommend you to see the pattern printing programs [Tricks] to develop the logic for almost all types of pattern programs in java. // Main Method. public static void main(String[] args) { // Initializing required number of lines. int n = 5;
Java Program to Print Inverted Triangle Alphabets Pattern
Write a Java program to print inverted triangle alphabets pattern using for loop. private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); . System.out.print("Enter Inverted Triangle of Alphabets Rows = "); int rows = sc.nextInt(); System.out.println("Printing Inverted Triangle Alphabets Pattern");
How to Print Alphabet Pattern in Java: A Step-by-Step Guide
Sep 5, 2023 · Learning how to print alphabet patterns in Java is not only a fundamental skill but also an excellent way to improve your coding abilities. In this article, we’ll walk you through the process of printing alphabet patterns in Java, from the basics to more complex patterns.
Alphabet Patterns — Java, Python, and C programming - Medium
Learn how to code different alphabet patterns in Java, Python, and C programs. 1. Pattern: Right-Angle Alphabet Triangle. For each and every row, we use an inner loop to print the...
Inverted Right Triangle Alphabet Pattern in Java: Pattern 1
Sep 29, 2021 · The programs below take the user input from the user for the number of rows and display the reverse right triangle character pattern in various character orders. This program can also be called the inverted half pyramid in java or Floyd’s triangle alphabet pattern in java.