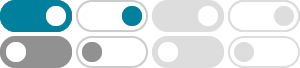
How do I put variables inside javascript strings? - Stack Overflow
First argument is the string that wanted to be parameterized. You should put your variables in this string like this format "%s1, %s2, ... %s12". Other arguments are the parameters respectively for that string.
How to interpolate variables in strings in JavaScript, without ...
var string = stringInject("this is a {0} string for {1}", ["test", "stringInject"]); which will replace the {0} and {1} with the array items and return the following string "this is a test string for stringInject" or you could replace placeholders with object keys and values like so:
How to insert variables in JavaScript strings? - Stack Overflow
Agreed, I'd expect it to be slower. However, my case was specific to referring to the same string literal hundreds of times per frame (say in a visualization with many dynamically labeled points). The speed hit of optimized lookup (which will just be indexing into a given offset) will likely not outweigh string creation time for each literal. I ...
String interpolation in JavaScript? - Stack Overflow
Jul 20, 2014 · While templates are probably best for the case you describe, if you have or want your data and/or arguments in iterable/array form, you can use String.raw. String.raw({ raw: ["I'm ", " years old!"] }, 3); With the data as an array, one can use the spread operator:
What does ${} (dollar sign and curly braces) mean in a string in ...
Mar 7, 2016 · Functionally, it looks like it allows you to nest a variable inside a string without doing concatenation using the + operator. I'm looking for documentation on this feature. I'm looking for documentation on this feature.
How to use a variable for a key in a JavaScript object literal?
In ES5 and earlier, you cannot use a variable as a property name inside an object literal. Your only option is to do the following: var thetop = "top"; // create the object literal var aniArgs = {}; // Assign the variable property name with a value of 10 aniArgs[thetop] = 10; // Pass the resulting object to the animate method <something>.stop ...
javascript - How can I refer to a variable using a string containing ...
In JavaScript, there is no standard for creating a 'Master Object', or any built-in method to access variables on the initial scope with a string reference that I am aware of. However, if you are using JavaScript for web development on a browser, the window Object will give you complete access to your current scope. For example:
Javascript Regex: How to put a variable inside a regular expression?
Per some of the comments, it's important to note that you may want to escape the variable if there is potential for malicious content (e.g. the variable comes from user input) ES6 Update. In 2019, this would usually be written using a template string, and the above code has been updated. The original answer was:
How to use a string as a variable name in Javascript?
May 28, 2011 · Possible Duplicates: Is there a way to access a javascript variable using a string that contains the name of the variable? JavaScript: Get local variable dynamicly by name string A simple cod...
Usage of the backtick character (`) in JavaScript
Dec 28, 2014 · In JavaScript, a backtick† ( ` ) seems to work the same as a single quote. For instance, I can use a backtick to define a string like this: var s = `abc`; Is there a way in which the behavior of the