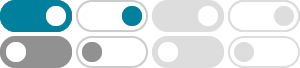
if statement - javascript if Null check - Stack Overflow
Dec 3, 2015 · JavaScript's OR operator doesn't return boolean true/false values like other languages. Rather it checks if the left evaluates to true when type casted to boolean, If true, it returns the value rather than a boolean. If false, then it …
How do I check for null values in JavaScript? - Stack Overflow
Jan 4, 2024 · To check for null SPECIFICALLY you would use this: if (variable === null) This test will ONLY pass for null and will not pass for "", undefined, false, 0, or NaN. Additionally, I've provided absolute checks for each "false-like" value (one that would return true for !variable).
javascript - How to check if a variable is not null? - Stack Overflow
Sep 5, 2017 · Here is how you can test if a variable is not NULL: if (myVar !== null) {...} the block will be executed if myVar is not null.. it will be executed if myVar is undefined or false or 0 or NaN or anything else..
JS Check for Null – Null Checking in JavaScript Explained
Nov 29, 2022 · Null is a primitive type in JavaScript. This means you are supposed to be able to check if a variable is null with the typeof () method. But unfortunately, this returns “object” because of an historical bug that cannot be fixed. let userName = null; ...
How to check for null, undefined or blank Variables in JavaScript
May 7, 2023 · In this article, we will explore the standards and best practices for checking for these types of variables in JavaScript. Null: A variable that is null has been explicitly set to have no value. Syntax: let v1 = null; typeof (var_); // "object"
How to Check for Null in JavaScript
Feb 12, 2025 · To determine whether a value is specifically null, the triple equality operator is the best method available. We can also use this in conjunction with Object.is() to determine a null value. Here’s a quick overview of both approaches.
How to Check if a Variable Is Null in JavaScript? An In-Depth …
In addition to if/else conditional checks, another clean way to check if a variable is null or undefined in JavaScript is by using the nullish coalescing operator ??.
Demystifying Null Checking in JavaScript: A Complete Guide to isnull
Nov 4, 2023 · Understanding null checking helps you write cleaner, more secure JavaScript code. By mastering the various techniques and best practices for isnull, you can handle those pesky null values with confidence!
javascript - Simplify checking "null or empty" for multiple …
Mar 21, 2017 · Solution: Then you can simply use the following condition: if (!string1 || !string2 || string1 === '' || string2 === ''){ //Your code here } Explanation: It will be true if one of the two strings is null or empty, in other words if any of the four conditions is true it will enter the if block.
How to check if a Variable Is Not Null in JavaScript
Sep 11, 2024 · Using the typeof operator, JavaScript checks if a variable is not null by verifying typeof variable !== ‘undefined’ && variable !== null. This approach ensures the variable exists and is not explicitly set to null, promoting reliable code execution.
- Some results have been removed