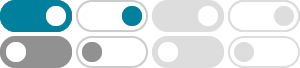
Heap Sort - Python - GeeksforGeeks
Mar 3, 2025 · The sort_heap( ) is an STL algorithm which sorts a heap within the range specified by start and end. Sorts the elements in the heap range [start, end) into ascending order. The second form allows you to specify a comparison function that determines when one element is less than another.
python - heapq with custom compare predicate - Stack Overflow
According to the heapq documentation, the way to customize the heap order is to have each element on the heap to be a tuple, with the first tuple element being one that accepts normal Python comparisons.
Heap Sort Algorithm in Python - Programming In Python
Apr 27, 2023 · In this tutorial, we’ve implemented Heap sort in Python by first writing a function to heapify a binary tree, and then using that function to implement Heap sort. We’ve also tested our implementation on a sample array and verified that it correctly sorts the array.
Python Heap Sort Program - Complete Guide with Examples
Learn how to implement Heap Sort in Python with this tutorial. Includes code examples for ascending and descending order sorting, and explanations for key heap operations.
Heap Sort Program in Python - Sanfoundry
Learn how to implement Heap Sort in Python with a detailed explanation, examples, time complexities, and runtime test cases.
How to Implement Heap Sort in Python - Delft Stack
Feb 2, 2024 · The implementation of the Heap sort algorithm in Python can be divided into two parts; a function that creates either a min-heap or a max-heap, and a function that implements the actual heap sort algorithm.
algorithm - Heap sort Python implementation - Stack Overflow
Feb 15, 2017 · for i in range(iterate,-1,-1): print "In %d iteration" %i. heapify(elements,i,size) print "heapified array is : " ,elements. #get input from user. nums = [6,9,3,2,4,1,7,5,10] #sort the list. heap_sort(nums)
Python Program for Heap Sort - Studytonight
Aug 17, 2021 · In this tutorial, we have performed a Heap Sort sort operation in python to sort an array. The heapsort algorithm doesn't have stability. The time complexity of the heapsort algorithm is O (n log n) and the space complexity is O (1). Want to learn coding? Over 20,000+ students enrolled.
Python Heap Sort Algorithm - CodersLegacy
In this article, we will be discussing the Python Heap Sort Algorithm in complete detail. We will start with it’s explanation, followed by a complete solution which is then explained by breaking it down into steps and explaining each of them separately.
Heap Sort Algorithm in Python – Learn Programming
Oct 9, 2024 · Heap sort is a comparison-based sorting algorithm that uses a binary heap data structure. It consists of two main phases: Building a max heap from the input data. Repeatedly extracting the maximum element from the heap and rebuilding the heap until all elements are sorted. largest = i # Initialize largest as root.