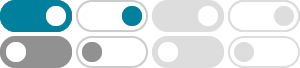
Python program to Sort a list according to the second element …
Dec 10, 2024 · The easiest way to sort a list by the second element of each sublist is by using the sorted () function with a lambda function as the key. The lambda function lets us specify which element in the sublist to focus on while sorting. Other methods that we can use to sort a list according to the second element of sublist in Python are:
sort nested list data in python - Stack Overflow
Jan 12, 2021 · I am trying to sort a nested list in python (3.8.5). I have a list like - ['2', 'B', 8, 15, 65, 20], ['3', 'C', 32, 35, 25, 140], ['4', 'D', 82, 305, 75, 90], ['5', 'E', 39, 43, 89, 55], and I want to sort like this - ['5', 'E', 39, 43, 89, 55], ['3', 'C', 32, 35, 25, 140], ['2', …
Sorting and Grouping Nested Lists in Python - Stack Overflow
Oct 24, 2008 · Use a function to reorder the list so that I can group by each item in the list. For example I'd like to be able to group by the second column (so that all the 21's are together) Lists have a built in sort method and you can provide a function that extracts the sort key.
Sorting a nested list in python - Stack Overflow
Apr 18, 2012 · You can pass the sorted() builtin a key argument - a function which takes the list item and returns the value to sort on. It's a little unclear exactly how you want to sort the list, you could clarify, although with this you should be able to work out a solution.
Sorting Nested Lists in Python – TheLinuxCode
Nov 4, 2023 · To sort a nested list based on an index of the sublists, use the key parameter along with a lambda function: nested_list = [[1, 5, 2], [3, 4], [6, 7, 8, 9]] nested_list.sort(key=lambda x: x[1]) print(nested_list) # Output: [[1, 2, 5], [6, 7, 8, 9], [3, 4]]
How to Sort Lists of Lists in Python with Examples
Jan 29, 2025 · In this guide, you’ll discover step-by-step methods for sorting lists of lists using Python’s built-in functions like sorted and list.sort(). We’ll also explore advanced techniques such as multi-criteria sorting, transforming data during sorting, and working with custom keys.
5 Best Ways to Sort a Python List of Lists by the Second Element
Feb 21, 2024 · This one-liner uses a nested list comprehension along with a temporary list of tuples for sorting. It’s concise but can be less readable for complex operations. Here’s an example: data_list = [[1, 3], [4, 2], [0, 1]] data_list = [x for _, x in sorted((sub[1], sub) for sub in data_list)] print(data_list) Output: [[0, 1], [4, 2], [1, 3]]
Sort List of Lists in Python – LivingWithCode
In Python, you can sort a nested list (list of lists) based on specific criteria using the sorted() function or the sort() method. Here’s how you can do it: To sort a nested list by the first element in each sublist, you can use the sorted() function or the sort() method.
5 Best Ways to Sort a List of Lists by Nth Element in Python
Feb 21, 2024 · The sorted() function in combination with a lambda function provides a clean, readable way to sort a list of lists by any nth element. The sorted() function returns a new sorted list, and the lambda function within it specifies the key to sort by – the nth element in this case. Here’s an example:
Sorting and Grouping Nested Lists in Python 3 - DNMTechs
Oct 4, 2024 · Sorting and grouping nested lists in Python 3 can be achieved using various techniques, such as using the sorted() function with a custom key function or the itertools.groupby() function. By combining these techniques, we can perform more complex operations on nested lists and manipulate data effectively.
- Some results have been removed