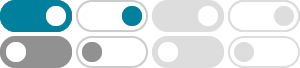
iteration - How to loop backwards in python? - Stack Overflow
def reverse(text): reversed = '' for i in range(len(text)-1, -1, -1): reversed += text[i] return reversed print("reverse({}): {}".format("abcd", reverse("abcd")))
Backward iteration in Python - GeeksforGeeks
Nov 20, 2024 · We can use slicing slicing notation [::-1] to reverse the order of elements in an iterable. This works for lists, tuples, and strings, but not for sets or dictionaries (since they are unordered). Using a while loop provides you control over the iteration process.
python - How do I reverse a list or loop over it backwards?
Oct 15, 2010 · Best solution is object.reverse() method; Create an iterator of the reversed list (because you are going to feed it to a for-loop, a generator, etc.) Best solution is reversed(object) which creates the iterator; Create a copy of the list, just …
loops - Traverse a list in reverse order in Python - Stack Overflow
Feb 10, 2009 · How do I traverse a list in reverse order in Python? So I can start from collection[len(collection)-1] and end in collection[0] . I also want to be able to access the loop index.
Reverse an Array in Python - 10 Examples - AskPython
Mar 10, 2020 · In this tutorial, we’ll go over the different methods to reverse an array in Python. The Python language does not come with array data structure support. Instead, it has in-built list structures that are easy to use as well as provide some methods to perform operations.
Reverse an Array upto a Given Position - GeeksforGeeks
Jan 20, 2025 · The numpy.flip() function reverses the order of array elements along the specified axis, preserving the shape of the array. Syntax: numpy.flip(array, axis) Parameters : array : [array_like]Array to be input axis : [integer]axis along which array is reversed. Returns : reversed array with shape prese
Reversing a List in Python - GeeksforGeeks
Oct 15, 2024 · If we want to reverse a list manually, we can use a loop (for loop) to build a new reversed list. This method is less efficient due to repeated insertions and extra space required to store the reversed list.
Reverse an Array in Python - Spark By Examples
May 30, 2024 · To iterate range (len (arr)-1, -1, -1) use for loop, it will return an array of elements in the reversing order. Related: In Python, you can use for loop to iterate iterable objects in reversing order and get them in backwards directions. Yields the below output.
Python For Loop in Backwards - Spark By Examples
May 30, 2024 · Pass range() function into reversed() function will reverse the range() function without any modification and then iterate reversed() function using for loop. This syntax will return the values in backward direction i.e. from back side.
Five different ways to reverse an array in python
Jul 15, 2021 · In python, we have some built-in methods to reverse an array it makes life easier, we can also use a slicing operator to reverse an array, or by using for-loop we can also perform...