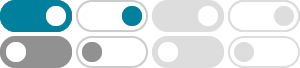
python - Pythonically add header to a csv file - Stack Overflow
If all you wanted to do was write an initial header, use a regular csv.writer() and pass in a simple row for the header: import csv with open('combined_file.csv', 'w', newline='') as outcsv: writer = csv.writer(outcsv) writer.writerow(["Date", "temperature 1", "Temperature 2"]) with open('t1.csv', 'r', newline='') as incsv: reader = csv.reader ...
How to add a header to a CSV file in Python? - GeeksforGeeks
Apr 5, 2025 · import pandas as pd # headers h = ["ID", "Name", "Department"] df = pd. read_csv ("gfg.csv", header = None, names = h) # Save the updated CSV file df. to_csv ("gfg_updated.csv", index = False) Output Explanation: pd.read_csv() load …
python - Append a Header for CSV file? - Stack Overflow
Jan 27, 2015 · i think you should use pandas to read the csv file, insert the column headers/labels, and emit out the new csv file. assuming your csv file is comma-delimited. something like this should work: from pandas import read_csv df = read_csv('test.csv') df.columns = ['a', 'b'] df.to_csv('test_2.csv')
How to import a csv file using python with headers intact, …
import csv with open("file.csv",'r') as f: reader = csv.reader(f) headers = next(reader) data = [{h:x for (h,x) in zip(headers,row)} for row in reader] #data now contains a list of the rows, with each row containing a dictionary # in the shape {header: value}.
How to add a header in a CSV file using Python?
Steps to add a header to a csv file using the pandas.DataFrame.to_csv() method are. Import the pandas module. Read the csv file as a pandas DataFrame object (pass header=None as an additional parameter).
Adding Headers to CSV Files in Python 3: A Pythonic Approach
Apr 18, 2024 · Here is a simple example that demonstrates how to add headers to a CSV file using the csv module in Python: import csv def add_headers_to_csv(file_path, headers): with open(file_path, 'r') as file: data = list(csv.reader(file)) with open(file_path, 'w', newline='') as file: writer = csv.writer(file) writer.writerow(headers) writer.writerows(data)
How to Read CSV with Headers Using Pandas? - AskPython
Sep 24, 2022 · Following is the syntax of read_csv (). df = pd.read_csv(“filename.txt”,sep=”x”, header=y, names=[‘name1’, ‘name2’…]) Where, filename.txt – name of the text file that is to be imported. x – type of separator used in the .csv file. Now we shall apply this syntax for importing the data from the text file shown earlier in this article.
How to Add a Header to a CSV File in Python? - Tpoint Tech
There are various ways to add a header to a CSV file in Python. Here are a few methods: When working with complex datasets in Python, using the Pandas library to add a header to a CSV file is convenient and efficient. Pandas makes working with tabular data easier by providing a high-level interface for data manipulation and analysis.
python - How to include headers into a csv file - Stack Overflow
Apr 23, 2014 · As Lutz Horn pointed out, you can use csv.writeheader if you're using py3.2+. If you're using py2.7 like me, maybe this might help: # Read the data. reader = csv.reader(csvfile, quoting=csv.QUOTE_NONE) writer = csv.writer(csvfile) writer.writerow(myHeaders) writer.writerows([row for row in reader])
Top 3 Methods to Efficiently Add Headers to CSV Files in Python
Nov 23, 2024 · Learn how to seamlessly add headers to your combined CSV files using Python with these top three methods.
- Some results have been removed