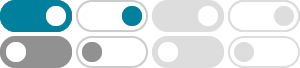
java - Postfix Evaluation using Stacks. - Stack Overflow
Aug 14, 2016 · System.out.println("Postfix Evauation = " + result); return result; Stacked st = new Stacked(100); //String y = new String("((z * j)/(b * 8) ^2"); String x = new String("2 2 2 * +"); TestingClass clas = new TestingClass(st); //clas.test(y);
Implement Stack using Array - GeeksforGeeks
Mar 21, 2025 · To implement a stack using an array, initialize an array and treat its end as the stack’s top. Implement push (add to end), pop (remove from end), and peek (check end) operations, handling cases for an empty or full stack.
Postfix evaluation in Java - CodeSpeedy
So let’s start learning postfix evaluation in Java. First of all, just create a stack that can store the values and operands of the expression. Check each expression one by one.
Java Stack Implementation using Array - HowToDoInJava
Mar 31, 2023 · This tutorial gives an example of implementing a Stack data structure using an Array. The stack offers to put new objects on the stack (push) and to get objects from the stack (pop).
Understanding Postfix-expression Evaluation in Java code using a stack
Jan 27, 2013 · The code is implementing used to read a postfix expression that only uses multiplication and addition. Then evaluate the expression while saving the results onto a stack.
java - Create stack to convert from infix to postfix using arrays ...
Oct 8, 2019 · First you need to create your "Stack" with a simple array. Like for example you could create a class StackArray with an array to back your elements. Then you need the methods that a Stack usually has (push, pop, peek...). Once you have your stack implementation you analyse your Infix-postfix-problem. My advice: do it on paper.
Java Implementation to evaluate a postfix expression using a stack
// Method to evaluate value of a postfix expression. static int evaluatePostfix(String exp) //create a stack. Stack<Integer> stack = new Stack<>(); // Scan all characters one by one. for(int i = 0; i < exp.length(); i++) char c = exp.charAt(i); if(c == ' ') continue; // If the scanned character is an operand. // (number here),extract the number.
Stack Implementation Using Array in Java - Java Guides
Implementing a stack using an array is straightforward, and it allows constant-time operations for push, pop, peek, isEmpty, and isFull. However, the main limitation is the array's fixed size, which requires careful consideration of the maximum size needed for the stack.
Stack: Data Structure — Implementation in Java using Array
Feb 27, 2023 · There are various ways to implementing a stack that includes using an array, linked list, array list and collection framework which is the easiest of all. Let’s take a closer look at the...
How to Implement Stack data structure in Java? Example Tutorial
Sep 27, 2023 · How to implement Your Own Stack in Java using an array? Example. Stack has following interface and supports operations like push (), pop (), contains (), clear () and size (). The pop () method will always return the last element added.