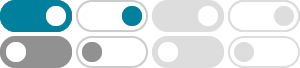
Search in 2D list using python to find x,y position
Aug 4, 2013 · If your "2D" list is rectangular (same number of columns for each line), you should convert it to a numpy.ndarray and use numpy functionalities to do the search. For an array of integers, you can use == for comparison.
Linear Search - Python - GeeksforGeeks
Apr 7, 2025 · The article explains how to find the index of the first occurrence of an element in an array using both iterative and recursive linear search methods, returning -1 if the element is not found.
Searching Algorithms for 2D Arrays (Matrix) - GeeksforGeeks
Apr 14, 2025 · In this article, we will explore three types of matrix search: Unsorted matrices, Completely sorted matrices, and Semi-sorted matrices (sorted row-wise, column-wise, or both). 1. Search in an Unsorted 2D Array. Examples: Input: mat[][] = [[77, 12, 9], [4, 1, 5], [6, 8, 3]] target = 5 Output: Element found in the matrix
Python | Using 2D arrays/lists the right way - GeeksforGeeks
Jun 20, 2024 · In this article, we are going to create a list of the numbers in a particular range provided in the input, and the other two lists will contain the square and the cube of the list in the given range using Python.
How to search for an element in a 2d array in Python
Jun 5, 2021 · This one is a linear search through a 2D array in Python. If I enter 11 as an argument, it finds it at position [0][0] - which is correct. However, if I change the argument to another number that is in the array, it returns 'The element has not been found.'
python - What is the fastest way of searching for string in 2d array ...
Feb 19, 2013 · Since the column is unsorted, linear search is going to be the fastest you can hope for from search, as you'll need to check every element. If this is a process you have to do repeatedly, you could create an index using a dictionary: index[list[i]] = index.get(list[i], list()) + [i]
Python Program For Linear Search (With Code & Explanation) - Python …
Linear search is a simple searching algorithm that traverses through a list or array in a sequential manner to find a specific element. In linear search, we compare each element of the list with the target element until we found a match or we have traversed the entire list.
Mastering 2D List Sorting and Searching in Python: A …
Searching for an element within a 2D list requires iterating through each row and column. The straightforward method is a linear search, checking each element one by one. If the matrix is sorted, more efficient algorithms like binary search can be applied per row or column. def binary_search_2d(matrix, target): for row in matrix: .
Linear Search in Python - Stack Abuse
Apr 18, 2024 · Linear Search, also known as Sequential Search, operates by traversing through the dataset, element by element until the desired item is found or the algorithm reaches the end of the collection. Its simplicity and ease of implementation make it a go-to choice for small datasets and lists where items are added or removed frequently.
5 Best Ways to Perform Linear Search on Lists or Tuples in Python
Mar 9, 2024 · Problem Formulation: In this article, we aim to provide efficient methods for performing a linear search on lists or tuples in Python. A linear search is the process of iterating through a list or tuple to find a specific element.
- Some results have been removed