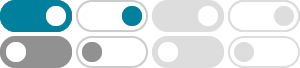
Python appending array to an array - Stack Overflow
Oct 31, 2016 · And what they wrote initially would do exactly what they wanted (assuming correct indentation): create a list and append two other lists to it.
How to declare and add items to an array in Python
Like C arrays, array.array objects hold only a single type (which has to be specified at object creation time by using a type code), whereas Python list objects can hold anything. It also defines append / extend / remove etc. to manipulate the data.
python - Add single element to array in numpy - Stack Overflow
When growing an array for a significant amount of samples it would be better to either pre-allocate the array (if the total size is known) or to append to a list and convert to an array afterward. Using np.append: b = np.array([0]) for k in range(int(10e4)): b = np.append(b, k) 1.2 s ± 16.1 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
python append to array in json object - Stack Overflow
Technically speaking, this is not a JSON object (even though it's formatted in the JSON style), it's a python dict. It's not "coming out right with brackets and quotes" because you are append() ing a string to the dictionary value [] .
python - Add numpy array as column to Pandas data frame
Here is other example: import numpy as np import pandas as pd """ This just creates a list of tuples, and each element of the tuple is an array""" a = [ (np.random.randint(1,10,10), np.array([0,1,2,3,4,5,6,7,8,9])) for i in range(0,10) ] """ Panda DataFrame will allocate each of the arrays , contained as a tuple element , as column""" df = pd.DataFrame(data =a,columns=['random_num','sequential ...
Appending two arrays together in Python - Stack Overflow
Nov 21, 2011 · I've been working in Python with an array which contains a one-dimensional list of values. I have until now been using the array.append(value) function to add values to the array one at a time. Now, I would like to add all the values from another array to the main array instead. In other words I don't want to add single values one at a time.
Addition of multiple arrays in python - Stack Overflow
newlist = [array([1,2,3,...5,4,3]), array([5,7,2,...4,6,7]), array([3,6,2,...4,5,9])] What would be the most pythonic way of getting a single array from the arrays in 'newlist' which is the addition of the arrays within it, such that (from newlist): sum = [8,15,7,...14,15,19] The arrays are …
How do I declare an array in Python? - Stack Overflow
Oct 3, 2009 · @AndersonGreen As I said there's no such thing as a variable declaration in Python. You would create a multidimensional list by taking an empty list and putting other lists inside it or, if the dimensions of the list are known at write-time, you could just write it as a literal like this: my_2x2_list = [[a, b], [c, d]].
python - Insert an element at a specific index in a list and return …
The cleanest approach is to copy the list and then insert the object into the copy. On Python 3 this can be done via list.copy: new = old.copy() new.insert(index, value) On Python 2 copying the list can be achieved via new = old[:] (this also works on Python 3). In terms of performance there is no difference to other proposed methods:
python - quick way to add values to a 2d array - Stack Overflow
Dec 13, 2013 · import numpy as Np # create an array with required shape. # Mind you cannot create an empty array. # Best way to create a zero array or ones array. shape_myarray=(3,3) x=Np.zeros(shape_myarray) y=Np.zeros(shape_myarray) # You can index through the numpy array to update it with any value.