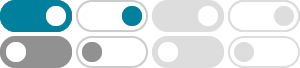
python - Trying to calculate the sum of numbers using while loop ...
Oct 26, 2018 · I'm trying to calculate the sum of multiple numbers using a while loop. When a negative number is entered the sum of the numbers should be printed. When I run the code all it does is print the last positive number entered. Here is the current non-working code: number = int(input('Enter a positive number: ')) if number > 0: tot = sum + number.
Python:How to make the sum of the values of a while loop store …
Aug 23, 2012 · I'm doing a tutorial about while loops on Codeacademy "Click here!" , but I've gotten stuck on this part: Write a while loop which stores into " theSum " the sum of the first 10 positive integers (including 10).This is what it gives you to work with: print num. num = num + 1. It prints out the numbers 1 to 10 on seperate lines in the console.
Python Program to Find the Sum of Natural Numbers Using While Loop
Jul 2, 2024 · In this example, a Python function sum_of_natural_numbers is defined to calculate the sum of the first N natural numbers using a while loop. The function initializes variables total and count, iterates through the numbers from 1 to N, and accumulates the sum in …
Sum of n natural numbers using while loop in python
Nov 22, 2021 · number = int (int (input ("Enter the number: ")) if number < 0: print("Enter a positive number: ") else: totalSum = 0 while (number > 0): totalSum += number number -= 1 print ("The sum is" , totalSum)
Print Sum of Numbers from 1 to 100 Using While Loop in Python
In this post we are going to discuss how you can find the sum of numbers between 1 to 100 using a while loop in Python: total += num. num += 1. # add the current number to the total. total += num # we can also use total = total + num. # increment the number by 1. num += 1. In the above code, we initialize the starting number and total it to 0.
How to sum in a For or a While Loop in Python | bobbyhadz
Apr 9, 2024 · To get the sum of N numbers using a while loop: Iterate for as long as the number is greater than 0. On each iteration, decrement the number by 1. On each iteration, increment the total by the number. sum_of_numbers += num. # 👇️ reassign num to num - 1 . num -= 1 print(sum_of_numbers) # 👉️ 15 (5 + 4 + 3 + 2 + 1) print(num) # 👉️ 0.
Python Program to Find Sum of First n Natural Numbers Using While Loop
In this tutorial, we are going to write a program to find the sum of the first n natural numbers using a while loop. In mathematics, the sum of the first n natural numbers is given by the formula n (n+1)/2.
How to Sum Numbers in For and While Loops in Python
This guide explores various techniques for calculating sums using both for and while loops in Python, covering different scenarios like summing items from a list, numbers in a range, and dynamically collecting values from user input.
Sum of n numbers using while loop in Python | Example code
Dec 23, 2021 · Simple use if statement with a while loop to calculate the Sum of n numbers in Python. Taken a number input from the user and stored it in a variable num. Use a while loop to iterate until num gets zero. In every iteration, add the num to the sum, and the value of the num is decreased by 1.
Sum of all Numbers between two given Numbers Using While Loop in Python
A while loop is used to iterate through all the numbers between the start and end (inclusive). At each iteration, the current number is added to the sum variable and the loop continues to the next number. Finally, the program prints the sum of the numbers using the print () function.