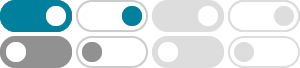
javascript - Sum two arrays in single iteration - Stack Overflow
It uses Array.prototype.reduce (), Array.prototype.map (), Math.max () and Array.from (): const n = arrays.reduce((max, xs) => Math.max(max, xs.length), 0); const result = Array.from({ length: n }); return result.map((_, i) => arrays.map(xs => xs[i] || 0).reduce((sum, x) => sum + x, 0));
Javascript: Multiply and Sum Two Arrays - Stack Overflow
Jul 29, 2011 · I have two arrays of equal length, and I need to multiply the corresponding (by index) values in each, and sum the results. For example. var arr1 = [2,3,4,5]; var arr2 = [4,3,3,1]; would result in 34 (4*2+3*3+4*3+5*1). What's the simplest to read way to write this?
JavaScript Array forEach() Method - W3Schools
The forEach() method calls a function for each element in an array. The forEach() method is not executed for empty elements.
javascript - Adding Consecutive Elements of Array - Stack Overflow
When i is 0 in your first array, the original would be adding 2 + 2 + 1 (numbers[i] + numbers[i] + 1) when you want 2 + 4 (numbers[i] + numbers[i+1]). Also when you do 'numbers.push(), you are adding an element to the numbers array. I think you want to do newArray.push() –
JavaScript – Add Array to Array of Array | GeeksforGeeks
Dec 2, 2024 · Here are the different methods to Array to an Array of Array (Multidimensional Array) in JavaScript. 1. Using push () Method. The push () method is one of the most common ways to add an array to an array of arrays. It adds a new element (in this case, an array) to the end of the array.
Array.prototype.forEach() - JavaScript | MDN - MDN Web Docs
Mar 13, 2025 · The forEach() method of Array instances executes a provided function once for each array element.
JavaScript: 6 Ways to Calculate the Sum of an Array
Feb 19, 2023 · This practical, succinct article walks you through three examples that use three different approaches to find the sum of all elements of a given array in Javascript (suppose this array only contains numbers).
javascript - How to append something to an array ... - Stack Overflow
Dec 9, 2008 · How do I append an object (such as a string or number) to an array in JavaScript? Use the Array.prototype.push method to append values to the end of an array: "Hi", "Hello", "Bonjour" You can use the push() function to append more than one value to an array in a single call: console.log(arr[i]);
How to Add the Numbers in a JavaScript Array - Expertbeacon
Aug 23, 2024 · In each iteration, the element of array (numbers[i]) is added to the sum variable using the += assignment operator. After the loop, sum contains the final result i.e. the total of all elements. This gets printed out.
Add Two Consecutive Elements from Array in JavaScript
Aug 31, 2020 · Learn how to add two consecutive elements from the original array and display the result in a new array using JavaScript with this detailed guide.
- Some results have been removed