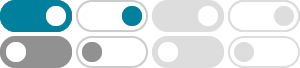
Python - Matrix - GeeksforGeeks
Apr 8, 2025 · In this tutorial, we’ll explore different ways to create and work with matrices in Python, including using the NumPy library for matrix operations. A Matrix is fundamentally a 2D list therefore we can create a Matrix by creating a 2D list (list of lists).
How to Create a Matrix in Python - Python Guides
Jun 3, 2024 · Learn how to create a matrix in Python using five methods like list of lists, numpy.array () function, matrix () function, nested for loop, and map () function with examples.
Python Matrix and Introduction to NumPy - Programiz
Access matrix elements. Similar like lists, we can access matrix elements using index. Let's start with a one-dimensional NumPy array. import numpy as np A = np.array([2, 4, 6, 8, 10]) print("A[0] =", A[0]) # First element print("A[2] =", A[2]) # Third element print("A[-1] =", A[-1]) # Last element
How can I create the following 4*4 Matrix from a 2*2 Matrix in Python?
May 15, 2020 · a = np.array ( [ [1,2], [3,4]]) b = np.array ( [ [1,2,1,2], [3,4,3,4], [1,2,1,2], [3,4,3,4]]) I want to convert a to b here. How can I do this? Thanks in advance.
NumPy Matrix Operations (With Examples) - Programiz
In NumPy, we use the np.array() function to create a matrix. For example, # create a 2x2 matrix . [5, 7]]) print("2x2 Matrix:\n",matrix1) # create a 3x3 matrix . [7, 14, 21], [1, 3, 5]]) print("\n3x3 Matrix:\n",matrix2) Output. [5 7]] [[ 2 3 5] [ 7 14 21] [ 1 3 5]]
Multidimensional Arrays in Python: A Complete Guide
Feb 27, 2023 · In this article, the creation and implementation of multidimensional arrays (2D, 3D as well as 4D arrays) have been covered along with examples in Python Programming language. To understand and implement multi-dimensional arrays in Python, the NumPy package is used.
Matrix manipulation in Python - GeeksforGeeks
Aug 7, 2024 · Let's discuss how to flatten a Matrix using NumPy in Python. By using ndarray.flatten() function we can flatten a matrix to one dimension in python. Syntax:numpy_array.flatten(order='C') order:'C' means to flatten in row-major.'F' means to flatten in column-major.'A' means to flatten in column-major
Mastering Matrices in Python: A Comprehensive Guide
Jan 26, 2025 · This blog aims to provide a detailed overview of matrices in Python, covering basic concepts, usage methods, common practices, and best practices. Table of Contents. Fundamental Concepts of Matrices in Python. What is a Matrix? Representing Matrices in Python; Usage Methods. Creating Matrices; Accessing Elements; Matrix Operations; Common ...
Matrix Operations in Python: A Comprehensive Guide
Jan 26, 2025 · In Python, performing matrix operations is made relatively straightforward with the help of powerful libraries. This blog aims to provide a detailed overview of matrix operations in Python, covering the basic concepts, how to use relevant …
Matrix Calculation in Python: A Comprehensive Guide
Jan 29, 2025 · Python, with its rich libraries and easy - to - use syntax, provides powerful tools for matrix calculations. In this blog, we will explore the basic concepts of matrix calculation in Python, how to use relevant libraries, common practices, and best practices.