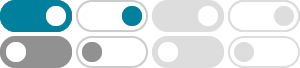
Python String Interpolation with the Percent (%) Operator
Aug 24, 2023 · The % operator tells the Python interpreter to format a string using a given set of variables, enclosed in a tuple, following the operator. A very simple example of this is as follows:
How do I print a '%' sign using string formatting?
Feb 5, 2015 · The new Python 3 approach is to use format strings. percent = 12 print("Percentage: {0} %\n".format(percent)) >>> Percentage: 12 % This is also supported in Python > 2.6. See the docs here: Python 3 and Python 2
Percentage Symbol (%) in Python
Nov 6, 2024 · The percent sign (%) in Python is used for string formatting and interpolation. It allows you to insert values into string templates using placeholders and formatting specifiers.
Python: % operator in print() statement - Stack Overflow
Dec 8, 2013 · The % operator in python for strings is used for something called string substitution. String and Unicode objects have one unique built-in operation: the % operator (modulo).
How can I selectively escape percent (%) in Python strings?
May 21, 2012 · If you are using Python 3.6 or newer, you can use f-string: >>> test = "have it break." >>> selectiveEscape = f"Print percent % in sentence and not {test}" >>> print(selectiveEscape) ... Print percent % in sentence and not have it break.
Python String Formatting – How to format String? - GeeksforGeeks
Aug 21, 2024 · There are five different ways to perform string formatting in Python. Formatting with % Operator. Formatting with format () string method. Formatting with string literals, called f-strings. Formatting with center () string method.
string — Common string operations — Python 3.13.3 …
3 days ago · class string.Formatter ¶ The Formatter class has the following public methods: format(format_string, /, *args, **kwargs) ¶ The primary API method. It takes a format string and an arbitrary set of positional and keyword arguments. It is just a wrapper that calls vformat().
Python - Output Formatting - GeeksforGeeks
Dec 16, 2024 · Python provides several ways to format strings effectively, ranging from old-style formatting to the newer f-string approach. The string modulo operator (%) is one of the oldest ways to format strings in Python. It allows you to embed values within a string by placing format specifiers in the string.
Python Modulo String Formatting - GeeksforGeeks
Mar 12, 2024 · In Python, a string of required formatting can be achieved by different methods. Some of them are; 1) Using % 2) Using {} 3) Using Template Strings In this article the formatting using % is discussed.
Python String Formatting
For new code, using str.format, or formatted string literals (Python 3.6+) over the % operator is strongly recommended. # "Hello Pete" We can use the %d format specifier to convert an int …