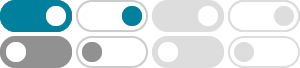
python - Check if string matches pattern - Stack Overflow
import re pat = re.compile(r'\A([A-Z][0-9]+)+\Z') pat.match('A1B2') # match pat.match('A1B2a') # no match This makes a difference if the string contains multiple lines and you want to match the pattern in latter lines in a string.
regex - Python regular expression not matching - Stack Overflow
Jul 16, 2013 · match only matches from the beginning of the string. Your code works fine if you do pct_re.search(line) instead. You should use re.findall instead: re.match will match at the start of the string. So you would need to build the regex for complete string. try this if …
Regex in Python. NOT matches - Stack Overflow
Mar 6, 2011 · As to removing the lines not matching this regex, you can simply construct a new string consisting of the lines that do match: new_s = "\n".join(line for line in s.split("\n") if re.match(regex, line))
re.match() in Python - GeeksforGeeks
Dec 16, 2024 · re.match method in Python is used to check if a given pattern matches the beginning of a string. It’s like searching for a word or pattern at the start of a sentence. For example, we can use re.match to check if a string starts with a certain word, number, or symbol.
Your Python Regex Pattern Doesn’t Match? Try This!
May 20, 2022 · A common reason why your Python regular expression pattern is not matching in a given string is that you mistakenly used re.match(pattern, string) instead of re.search(pattern, string) or re.findall(pattern, string).
Pattern matching in Python with Regex - GeeksforGeeks
Jul 6, 2024 · Following regex is used in Python to match a string of three numbers, a hyphen, three more numbers, another hyphen, and four numbers. Any other string would not match the pattern. Regular expressions can be much more sophisticated. For example, adding a 3 in curly brackets ( {3}) after a pattern is like saying, “Match this pattern three times.”
re — Regular expression operations — Python 3.13.3 …
1 day ago · A regular expression (or RE) specifies a set of strings that matches it; the functions in this module let you check if a particular string matches a given regular expression (or if a given regular expression matches a particular string, which comes down to the same thing).
Python RegEx - W3Schools
RegEx can be used to check if a string contains the specified search pattern. Python has a built-in package called re, which can be used to work with Regular Expressions. Import the re module: When you have imported the re module, you can start using regular expressions: Search the string to see if it starts with "The" and ends with "Spain":
Python re.match - Mastering Regular Expression Matching
1 day ago · Unlike re.search, which looks anywhere in the string, re.match only checks the start. It returns a match object if found or None otherwise. This function is useful for validating input formats or extracting data from structured text where patterns appear at the beginning. Basic Syntax. The syntax for re.match is straightforward: re.match ...
Regular Expression HOWTO — Python 3.13.3 documentation
1 day ago · If you’re matching a fixed string, or a single character class, and you’re not using any re features such as the IGNORECASE flag, then the full power of regular expressions may not be required. Strings have several methods for performing operations with fixed strings and they’re usually much faster, because the implementation is a single ...