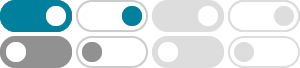
JavaScript Program to Check Prime Number
Write a function to check if a number is prime or not. A number is prime if it has only two distinct divisors: 1 and itself. For example, 3 is a prime number because it has only two distinct divisors: 1 and 3. Return "Prime" if num is prime; otherwise, return …
Check Number prime in JavaScript - Stack Overflow
function isPrime(number) { if((number % 2 === 0 && number !== 2) || number <= 1) { return false; } const limit = Math.floor(Math.sqrt(number)); for(let index = 3; index <= limit; index += 2) { if (number % index === 0) { return false; } } return true; }
JavaScript Program to Print Prime Numbers from 1 to N
Feb 19, 2024 · In this article, we'll explore how to create a JavaScript program to print all prime numbers from 1 to a given number N. To find prime numbers from 1 to N, we need to: Iterate through all numbers from 2 to N (since 1 is not a prime number). For each number, check if it is divisible by any number other than 1 and itself.
Check Prime Number in JavaScript (5 Programs) - WsCube Tech …
Jan 24, 2024 · The program then checks if the entered number is prime using the isPrime function. Depending on the result, it prints either “[number] is a prime number.” or “[number] is not a prime number.”, with [number] being the user-entered number.
4 ways in JavaScript to find if a number is prime or not
Jun 8, 2023 · In this post, I will show you how to check if a number is prime or not in JavaScript with examples. Method 1: By using a for loop: This is the simplest way to find a prime number.
Check a Number is Prime or Not Using JavaScript
6 days ago · To check if a number is prime, you can use different methods, such as checking divisibility up to the square root for efficiency or using the Sieve of Eratosthenes for multiple numbers. The optimized square root method is usually the …
Javascript Program for Prime Numbers - GeeksforGeeks
Aug 28, 2024 · In this article, we'll explore how to create a JavaScript program to print all prime numbers from 1 to a given number N. To find prime numbers from 1 to N, we need to: Iterate through all numbers 3 min read
JavaScript Program to Check if a Number is Prime - Java Guides
This JavaScript program demonstrates how to check whether a number is prime by checking divisibility. The algorithm efficiently reduces the number of checks by only testing up to the square root of the number, making it faster for larger numbers.
javascript - Programming to find if a number is prime - Stack Overflow
if (total % l == 0) {prime = "this is not a prime number"} else {prime = "this is a prime number"} the code is used in a html file as part of a function. You need to break out of the loop as soon as you discover the number isn't prime.
JavaScript: Check a number is prime or not - w3resource
Feb 28, 2025 · Write a JavaScript function that checks if a number is prime using recursion without employing any loops. Write a JavaScript function that recursively tests for factors and returns a boolean indicating whether the number is prime.
- Some results have been removed