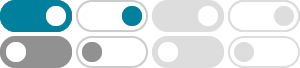
Python Program to Display Fibonacci Sequence Using Recursion
In this program, you'll learn to display Fibonacci sequence using a recursive function.
Fibonacci Series in Python using Recursion - Python Examples
In this tutorial, we present you two ways to compute Fibonacci series using Recursion in Python. The first way is kind of brute force. The second way tries to reduce the function calls in the recursion.
algorithm - Fast Fibonacci recursion - Stack Overflow
You can do a pretty fast version of recursive Fibonacci by using memoization (meaning: storing previous results to avoid recalculating them). for example, here's a proof of concept in Python, where a dictionary is used for saving previous results:
A Python Guide to the Fibonacci Sequence – Real Python
In this step-by-step tutorial, you'll explore the Fibonacci sequence in Python, which serves as an invaluable springboard into the world of recursion, and learn how to optimize recursive algorithms in the process.
Python Data Structures and Algorithms - Recursion: Fibonacci sequence
Mar 27, 2025 · Write a Python program to implement a recursive Fibonacci function with memoization to optimize performance. Write a Python program to recursively compute the Fibonacci sequence and print each term as it is generated.
How to calculate fibonacci Series Using Recursion? - codedamn
Mar 10, 2024 · To implement the Fibonacci series using recursion, we start by defining a function that accepts an integer n as its parameter. The function then checks if n is either 0 or 1, the base cases, returning n itself.
Python Program To Print Fibonacci Series Using Recursion
In this tutorial, you will learn to write a Python Program To Print Fibonacci Series Using Recursion. The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding numbers. The first two numbers in the series are 0 and 1, and the rest of the series is generated by adding the previous two numbers.
Fibonacci Series in Python - Sanfoundry
In Python, the Fibonacci series can be implemented using a loop or recursion. It starts with 0 and 1, and each subsequent number is the sum of the two previous numbers. For example, the Fibonacci series begins as 0, 1, 1, 2, 3, 5, 8, 13, and so on. Mathematically, we can denote it as: Fn = Fn-1 + Fn-2.
Generate Fibonacci Series in Python - PYnative
Mar 27, 2025 · Explanation: The function initializes a and b with the first two Fibonacci numbers.; A while loop continues as long as the count is less than n.; Inside the loop, the next Fibonacci number is calculated and appended to the list. a and b are updated to prepare for the next iteration.; 3. Using Recursion. Recursion is a programming technique where a function calls itself to solve smaller ...
Fibonacci Series Using Recursion - Online Tutorials Library
Let us learn how to create a recursive algorithm Fibonacci series. The base criteria of recursion. declare f0, f1, fib, loop . set f0 to 0 . set f1 to 1 . display f0, f1. for loop ← 1 to n. fib ← f0 & plus; f1 . f0 ← f1. f1 ← fib. display fib. end for .