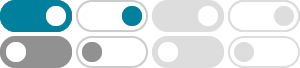
Java Program for QuickSort - GeeksforGeeks
Jan 31, 2025 · Program to Implement Quick Sort in Java. Complexity of the above Program: Auxiliary Space: O (N) in the worst case and O (Log n) on average. Mainly the extra space required is for recursion call stack and the worst case happens when one part is always empty. Please refer complete article on QuickSort for more details!
Java Program to Implement Quick Sort Algorithm
Quicksort algorithm is based on the divide and conquer approach where an array is divided into subarrays by selecting a pivot element. In this example, we will implement the quicksort algorithm in Java.
Quicksort Algorithm Implementation in Java - Baeldung
May 30, 2024 · In this tutorial, we’ll explore the QuickSort algorithm in detail, focusing on its Java implementation. We’ll also discuss its advantages and disadvantages and then analyze its time complexity.
Quick Sort Algorithm in Java
Let's create a generic implementation of the Quick Sort algorithm so that we can sorting Integer, String, Double etc. This program sorts according to the natural ordering of its elements.
QuickSort In Java - Algorithm, Example & Implementation
Apr 1, 2025 · This Tutorial Explains the Quicksort Algorithm in Java, its illustrations, QuickSort Implementation in Java with the help of Code Examples.
Quick Sort Program in Java - Sanfoundry
Quick Sort is a sorting algorithm used in Java that employs a divide-and-conquer strategy to sort a list of elements. It works by selecting a “pivot” element from the array and then partitioning the other elements into two sub-arrays based on whether they are less than or greater than the pivot.
Quicksort Java algorithm - Examples Java Code Geeks - 2025
Jun 19, 2014 · First, we are going to explain how Quick sort works on an algorithmic level, with some simple examples. Finally, we will build our implementation in Java and discuss its performance.
QuickSort in Java: A Fast and Efficient Sorting Algorithm
Jul 31, 2024 · Introduction In this article, we will explore how QuickSort works and implement it in Java. QuickSort is one of the most popular and efficient sorting algorithms used in computer science. It's known for its speed and is widely used in …
Java quick sort algorithm - W3schools
Quicksort is a divide and conquer algorithm. Quicksort first divides a large array into two smaller sub-arrays: the low elements and the high elements. Quicksort can then recursively sort the sub-arrays. Pick an element, called a pivot, from the array.
QuickSort Complete Tutorial | Example | Algorithm | Programming …
Dec 3, 2023 · How to implement the QuickSort algorithm? The below function can be used as a recursive approach to sort elements using Quick Sort. if(start >= end){ //calling partition function. pIndex <- Partition(A,start,end); . QuickSort(A, start, pIndex-1); QuickSort(A,pIndex+1,end);