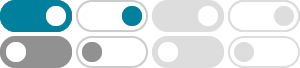
Split a string by a delimiter in Python - Stack Overflow
When you want to split a string by a specific delimiter like: __ or | or , etc. it's much easier and faster to split using .split() method as in the top answer because Python string methods are …
In Python, how do I split a string and keep the separators?
Here is a simple .split solution that works without regex.. This is an answer for Python split() without removing the delimiter, so not exactly what the original post asks but the other …
Split a string by backslash in python - Stack Overflow
You can split a string by backslash using a.split('\\'). The reason this is not working in your case is that \x in your assignment a = "1\2\3\4" is interpreted as an octal number. If you prefix the …
How do i split a string in python with multiple separators?
Jun 15, 2012 · Return a list of the words in the string, using sep as the delimiter string. If maxsplit is given, at most maxsplit splits are done (thus, the list will have at most maxsplit+1 elements). …
How does Python split () function works - Stack Overflow
Dec 4, 2016 · For example \n is a newline character inside a python string which will lose its meaning in a raw string and will simply mean backslash followed by n. string.split() string.split() …
How to split by comma and strip white spaces in Python?
$ string = "blah, lots , of , spaces, here " $ re.split(', ',string) ['blah', 'lots ', ' of ', ' spaces', 'here '] This doesn't work well for your example string, but works nicely for a comma-space separated …
How to split a Python string on new line characters
The main difference between Python 2.X and Python 3.X is that the former uses the universal newlines approach to splitting lines, so "\r", "\n", and "\r\n" are considered line boundaries for 8 …
python - Splitting on first occurrence - Stack Overflow
Using .split >>> s.split('mango', 1) ['123', ' abcd mango kiwi peach'] The second parameter to .split limits the number of times the string will be split. This gives the parts both before and after the …
Dividing a string at various punctuation marks using split()
Mar 21, 2012 · Using string methods, itertools.groupby, and actually writing functions (!), some of us manage to get by almost never using regexes, and in exchange for a few more keystrokes …
python - How to split a dataframe string column into two columns ...
Jan 15, 2018 · 1: If you're unsure what the first two parameters of .str.split() do, I recommend the docs for the plain Python version of the method. But how do you go from: a column containing …