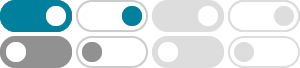
Python list vs. array – when to use? - Stack Overflow
Aug 17, 2022 · array.array is also a reasonable way to represent a mutable string in Python 2.x (array('B', bytes)). However, Python 2.6+ and 3.x offer a mutable byte string as bytearray. However, if you want to do math on a homogeneous array of numeric data, then you're much better off using NumPy, which can automatically vectorize operations on complex ...
How do I declare an array in Python? - Stack Overflow
Oct 3, 2009 · @AndersonGreen As I said there's no such thing as a variable declaration in Python. You would create a multidimensional list by taking an empty list and putting other lists inside it or, if the dimensions of the list are known at write-time, you could just write it as a literal like this: my_2x2_list = [[a, b], [c, d]].
How to create an integer array in Python? - Stack Overflow
Dec 7, 2009 · Use the array module. With it you can store collections of the same type efficiently. >>> import array >>> import itertools >>> a = array_of_signed_ints = array.array("i", itertools.repeat(0, 10)) For more information - e.g. different types, look at the documentation of the array module. For up to 1 million entries this should feel pretty snappy.
python - How to define a two-dimensional array? - Stack Overflow
Jul 12, 2011 · I want to define a two-dimensional array without an initialized length like this: Matrix = [][] But this gives an error: IndexError: list index out of range
python - How to modify list entries during for loop? - Stack Overflow
Sep 8, 2023 · But in Python they are not mutable. Any string operation returns a new string. If you had a list of objects you knew were mutable, you could do this as long as you don't change the actual contents of the list.
python - How do I create a list with numbers between two values ...
Aug 16, 2013 · In python you can do this very eaisly start=0 end=10 arr=list(range(start,end+1)) output: arr=[0,1,2,3,4,5,6,7,8,9,10] or you can create a recursive function that returns an array upto a given number:
Colon (:) in Python list index - Stack Overflow
I'm new to Python. I see : used in list indices especially when it's associated with function calls. Python 2.7 documentation suggests that lists.append translates to a[len(a):] = [x]. Why does one need to suffix len(a) with a colon? I understand that : is used to identify keys in dictionary.
python - array.array versus numpy.array - Stack Overflow
Jun 21, 2022 · In defense of array.array, I think its important to note that it is also a lot more lightweight than numpy.array, and that saying 'will do just fine' for a 1D array should really be 'a lot faster, smaller, and works in pypy/cython without issues.'
python - How to remove specific elements in a numpy array
Jun 12, 2012 · Using np.delete is the fastest way to do it, if we know the indices of the elements that we want to remove. . However, for completeness, let me add another way of "removing" array elements using a boolean mask created with the help of np
python - How can I access the index value in a 'for' loop? - Stack …
Tested on Python 3.12. Here are twelve examples of how you can access the indices with their corresponding array's elements using for loops, while loops and some looping functions. Note that array indices always start from zero by default (see example 4 to change this). 1. Looping elements with counter and += operator.