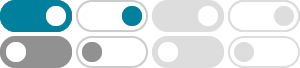
How to get exception message in Python properly
Oct 20, 2015 · What is the best way to get exceptions' messages from components of standard library in Python? I noticed that in some cases you can get it via message field like this: try: pass except Exception as ex: print(ex.message) but in some cases (for example, in case of socket errors) you have to do something like this: try: pass except socket.error ...
How do I print an exception in Python? - Stack Overflow
Jun 20, 2022 · If you are going to print the exception, it is better to use print(repr(e)); the base Exception.__str__ implementation only returns the exception message, not the type. Or, use the traceback module, which has methods for printing the …
Manually raising (throwing) an exception in Python
Jun 13, 2022 · Use the most specific Exception constructor that semantically fits your issue. Be specific in your message, e.g.: raise ValueError('A very specific bad thing happened.') Avoid raising a generic Exception. To catch it, you'll have to catch all other more specific exceptions that subclass it. raise Exception('I know Python!') # Don't!
Python Try Except: Examples And Best Practices
Sep 24, 2024 · In this article, you will learn how to handle errors in Python by using the Python try and except keywords. It will also teach you how to create custom exceptions, which can be used to define your own specific error messages.
8. Errors and Exceptions — Python 3.13.3 documentation
3 days ago · If an exception occurs which does not match the exception named in the except clause, it is passed on to outer try statements; if no handler is found, it is an unhandled exception and execution stops with an error message.
Python Try and Except Statements – How to Handle Exceptions in Python
Sep 23, 2021 · In this tutorial, you've learned how you can use try and except statements in Python to handle exceptions. You coded examples to understand what types of exception may occur and how you can use except to catch the most common errors.
Python Try Except - W3Schools
The try block lets you test a block of code for errors. The except block lets you handle the error. The else block lets you execute code when there is no error. The finally block lets you execute code, regardless of the result of the try- and except blocks.
Python Try Except - GeeksforGeeks
Mar 19, 2025 · Python provides try and except blocks to handle situations like this. In case an error occurs in try-block, Python stops executing try block and jumps to exception block.
Try and Except in Python - Python Tutorial
Python has built-in exceptions which can output an error. If an error occurs while running the program, it’s called an exception. If an exception occurs, the type of exception is shown. Exceptions needs to be dealt with or the program will crash. To handle exceptions, the try …
Python Print Exception – How to Try-Except-Print an Error
Mar 15, 2023 · Python comes with a built-in try…except syntax with which you can handle errors and stop them from interrupting the running of your program. In this article, you’ll learn how to use that try…except syntax to handle exceptions in your code so they don’t stop your program from running. What is an Exception? In Python, an exception is an error object.
- Some results have been removed