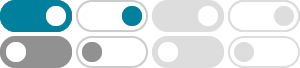
C++ Program To Find Transpose of a Matrix - GeeksforGeeks
Jun 21, 2023 · Transpose of a matrix is obtained by changing rows to columns and columns to rows. In other words, the transpose of A [] [] is obtained by changing A [i] [j] to A [j] [i]. Example: 1. For Square Matrix. The below program finds the transpose of A [] [] and stores the result in B [] [], we can change N for a different dimension. 1 2 3 4.
Transposing 2D array in C++ - Stack Overflow
In-place transpose: void transpose(vector<vector<int>>& matrix) { // assumes square matrix int wh = matrix.size(); for(int y=0; y < wh; ++y) { for(int x=0; x < y; ++x) { swap(matrix[y][x], matrix[x][y]); } } }
What is the fastest way to transpose a matrix in C++?
May 24, 2013 · For a C++ project, you can make use of the Armadillo C++: #include <armadillo> void transpose( arma::mat &matrix ) { arma::inplace_trans(matrix); }
Program to find transpose of a matrix - GeeksforGeeks
Feb 26, 2025 · Given a matrix of size n X m, find the transpose of the matrix. Transpose of a matrix is obtained by changing rows to columns and columns to rows. In other words, transpose of mat[n][m] is obtained by changing mat[i][j] to mat[j][i]. Example: Approach using (N^2) space for …
C++ Program to Transpose the Matrix (2-D Array)
May 24, 2023 · In this tutorial, we’ll learn about the transpose of matrices (2-D Array) i.e. a program to transpose the matrix using the C++ programming language. Transpose of matrix means exchanging the rows into column and column into rows.
Inplace (Fixed space) M x N size matrix transpose
Aug 15, 2023 · If the matrix is symmetric in size, we can transpose the matrix inplace by mirroring the 2D array across it’s diagonal (try yourself). How to transpose an arbitrary size matrix inplace? See the following matrix, As per 2D numbering in C/C++, corresponding location mapping looks like, 0 a 0. 1 b 4. 2 c 8. 3 d 1.
c - Transpose of a Matrix 2D array - Stack Overflow
Jan 10, 2017 · As there must be only one array in the program, a valid approach would be to transpose the matrix in-place, which can be done with the following nested loops. for( int i = 0; i < n; i++) { for ( j = i+1; j < n; j++ ) // only the upper is iterated { swap(&(a[i][j]), &(a[j][i])); } }
Write a program to find the transpose of a 2D array.
Oct 21, 2024 · One essential operation involving matrices is finding the transpose of a 2D array. The transpose of a matrix is formed by swapping its rows with columns, resulting in a new matrix. In this article, we will explore the concept of finding …
Efficient Matrix Transposition in C++: A Step-by-Step Guide
Sep 9, 2024 · Given an n x m matrix, the task is to return its transpose. The transpose of a matrix is obtained by flipping it over its diagonal, which means converting the matrix's rows into columns and vice...
C++ Program to Find Transpose of a Matrix
This program takes a matrix of order r*c from the user and computes the transpose of the matrix.