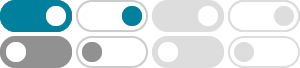
Fibonacci: Top-Down vs Bottom-Up Dynamic Programming
Mar 18, 2024 · In this article, we covered how to compute numbers in the Fibonacci Series with a recursive approach and with two dynamic programming approaches. We also went over the pseudocode for these algorithms and discussed their time and space complexity.
Fibonacci Sequence using Dynamic Programming - AlgoDaily
Time Complexity: The bottom-up dynamic programming approach has a time complexity of O(n), where n is the index of the Fibonacci number we want to compute. This is because we only need to perform n computations to calculate the desired Fibonacci number.
Solving Fibonacci Numbers using Dynamic Programming
Nov 29, 2020 · The time complexity of both the memoization and tabulation solutions are O(n) — time grows linearly with the size of n, because we are calculating fib(4), fib(3), etc each one time. Note:...
Computational complexity of Fibonacci Sequence - Stack Overflow
Aug 10, 2017 · In addition, you can find optimized versions of Fibonacci using dynamic programming like this: static int fib(int n) { /* memory */ int f[] = new int[n+1]; int i; /* Init */ f[0] = 0; f[1] = 1; /* Fill */ for (i = 2; i <= n; i++) { f[i] = f[i-1] + f[i-2]; } return f[n]; }
Computational Complexity of Fibonacci Sequence - Baeldung
Mar 18, 2024 · In this article, we analyzed the time complexity of two different algorithms that find the n th value in the Fibonacci Sequence. First, we implemented a recursive algorithm and discovered that its time complexity grew exponentially in n .
algorithm - What is the time complexity of computing the Nth fibonacci …
Jul 6, 2020 · I recently solved the time complexity for the Fibonacci algorithm using recursion. This is a standard solution with a time complexity of O(2^n). I was wondering if you were to use a DP algorithm, which saves fibonacci numbers that have already been calculated, what …
Fibonacci using Dynamic Programming in Java - JavaCodeMonk
Nov 21, 2020 · Time complexity of an algorithm is Big O (2 n) i.e. exponential when its growth doubles with each addition to the input data set. It is exactly opposite to Big O (log n) where problem size is reduced to half on each successive iteration.
Recursion vs Dynamic Programming – Fibonacci(Leetcode 509)
Oct 3, 2021 · The red line represents the time complexity of recursion, and the blue line represents dynamic programming. The x-axis means the size of n. And y-axis means the time the algorithm will consume in order to compute the result.
Dynamic Programming Fibonacci algorithm - Stack Overflow
Iterative solution to find the nth fibonnaci takes O (n) in terms of the value of n and O (2^length (n)) in terms of the size of n ( length (n) == number of bits to represent n). This kind of running time is called Pseudo-polynomial.
Introduction To Dynamic Programming - Fibonacci Series
Time Complexity: T(n) = T(n-1) + T(n-2) + 1 = 2 n = O(2 n) Use Dynamic Programming - Memoization: Store the sub-problems result so that you don't have to calculate again. So first check if solution is already available, if yes then use it else calculate and store it for future.
- Some results have been removed