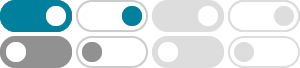
Python Program to Swap Two Variables
# Python program to swap two variables x = 5 y = 10 # To take inputs from the user #x = input('Enter value of x: ') #y = input('Enter value of y: ') # create a temporary variable and swap the values temp = x x = y y = temp print('The value of x after swapping: {}'.format(x)) print('The value of y after swapping: {}'.format(y))
Python Swap Two Numbers - PYnative
Mar 27, 2025 · In this tutorial, we covered multiple ways to swap two numbers in Python: Using a temporary variable; Without a temporary variable (arithmetic operations) Using tuple unpacking; Using XOR bitwise operator; Swapping in a function; Using collections like lists; Each method has its own advantages.
Python Program For Swapping Of Two Numbers (4 Methods) - Python …
Swapping two numbers in Python is a fundamental operation that allows us to manipulate and rearrange data efficiently. In this article, we explored different methods for swapping two numbers: using a temporary variable, arithmetic operations, tuple unpacking, and XOR operations.
How to Swap Two Numbers in Python Using Function [4 Examples]
Feb 7, 2024 · In this Python tutorial, I will explain the various methods to swap two numbers in Python using function, i.e., with arguments and return value, with arguments and without return value, without arguments and with return value, and without arguments and return value.
Swapping of Two Numbers in Python - Python Guides
Apr 23, 2024 · In this Python tutorial, you will learn multiple ways to swap two numbers in Python using practical examples and realistic scenarios. While working on a Python Project, I needed to sort the data, so I used some sorting algorithms that …
Python Program to Swap Two Numbers - Tutorial Gateway
Write a Program to Swap Two Numbers in Python using the Temp variable, Bitwise Operators, and Arithmetic Operators. The standard technique is using temporary variables. However, Python allows you to assign values to multiple variables using a comma, the best alternative method.
Python Program to Swap Two Numbers - Example Project
Aug 17, 2023 · This Python program takes two numbers as input and swaps their values using tuple assignments. The program then displays the numbers before and after swapping. Code:
Python Program to Swap Two Numbers - BeginnersBook
Jun 8, 2018 · In this tutorial we will see two Python programs to swap two numbers. In the first program we are swapping the numbers using temporary variable and in the second program we are swapping without using temporary variable.
Different ways to Swap two numbers in Python – allinpython.com
learn 5 different ways to Swap two numbers in python with a detailed explanation. 1) using Comma Operator 2) using Arithmetic Operator (+,-) , 3) XOR Operator..
Python Program to Swap Two Variables - GeeksforGeeks
Feb 21, 2025 · The task of swapping two numbers without using a third variable in Python involves taking two input values and exchanging their values using various techniques without the help of a temporary variable.