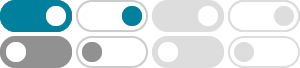
sum of two numbers coming from the command line
Here is the program to take two numbers as command-line arguments (while invoking the script) and display sum (using python): import sys a= sys.argv[1] b= sys.argv[2] sum=str( a+b) print " sum is", sum
Python Program to Add two Numbers using Command Line Arguments
In this article, we will learn about python program to add two numbers using command line arguments. Getting Started. The task is write a python program that returns sum of two numbers provided as command line arguments. sys.argv reads input from common line. It is present in sys module in python.
command line arguments to add two numbers in python
Sep 25, 2017 · import sys if len(sys.argv) > 2: try: a = int(sys.argv[1]) b = int(sys.argv[2]) print("sum = %d" % (a + b)) except ValueError: print("failed to parse all arguments as integers.") exit(1) else: print("Not enough numbers to add")
Command-Line Option and Argument Parsing using argparse in Python
Aug 14, 2024 · This code utilizes the ‘argparse' module to create a command-line interface for calculating the average of floating-point numbers. It defines two command-line arguments: ‘integers’ to accept multiple floating-point values and ‘sum’ and ‘len’ to …
Command Line Arguments in Python - GeeksforGeeks
Mar 17, 2025 · Example: Let’s suppose there is a Python script for adding two numbers and the numbers are passed as command-line arguments. Output: Python getopt module is similar to the getopt () function of C. Unlike sys module getopt module extends the separation of the input string by parameter validation.
python - Function to sum multiple numbers - Stack Overflow
Apr 26, 2018 · You want to have an *args parameter in your function so that you may take as many inputs more than 1: total = num1. for num in args: total = total + num. return total. What the *args means is you can pass through as many arguments as you want:
Python Script Arguments: Unleashing the Power of Dynamic …
6 days ago · Here's an example of a script that uses argparse to calculate the sum of two numbers: # sum_numbers_argparse.py import argparse parser = argparse.ArgumentParser(description='Calculate the sum of two numbers') parser.add_argument('number1', type=float, help='The first number') parser.add_argument('number2', type=float, help='The second number ...
How to Add Two Numbers in Python? - Python Guides
Nov 4, 2024 · To add two numbers in Python, you can define a simple function that takes two parameters and returns their sum. For example: def add_two_numbers(number1, number2): return number1 + number2 result = add_two_numbers(5, 10) print(result) # Output: 15
Command line arguments · Python Basics
sys.exit("Error: Please provide exactly two numbers as arguments") else: (num1, num2) = sys.argv[1:] total = int(num1) + int(num2) print("{} + {} = {}".format(num1, num2, total)) $ echo $? File "./sum_of_two_numbers.py", line 9, in <module> total = int(num1) + int(num2) $ echo $? if len(sys.argv) < 2:
Python Program to Add Two Numbers
In the program below, we've used the + operator to add two numbers. Example 1: Add Two Numbers # This program adds two numbers num1 = 1.5 num2 = 6.3 # Add two numbers sum = num1 + num2 # Display the sum print('The sum of {0} and {1} is {2}'.format(num1, num2, sum)) Output. The sum of 1.5 and 6.3 is 7.8. The program below calculates the sum of ...
- Some results have been removed