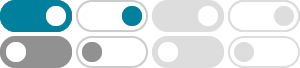
python - Store a value in an array inside one for loop - Stack Overflow
Feb 2, 2023 · In simplest way, to store the value of for loop in array. from array import * ar = [] for i in range (0, 5, 1) : x = 3.0*i+1 ar.append(x) print(ar) Output like this; [1.0, 4.0, 7.0, 10.0, 13.0]
Python Arrays - W3Schools
Arrays are used to store multiple values in one single variable: Create an array containing car names: What is an Array? An array is a special variable, which can hold more than one value at a time. If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
Store multiple values in arrays Python - Stack Overflow
Nov 24, 2018 · You could use arrays[len(arrays[0]) - len(arrays[1])].append(element) in order to decouple from element. Well, or simply enumerate(lst). –
python - How to store results of for loop as an array ... - Stack Overflow
Jun 25, 2019 · import numpy as np # same as before def celsius2fahrenheit(x): return (x * 1.8 + 32) # allocate the memory pair_arr = np.zeros(2, 35) for i in range(35): # generate a random number x = np.random.randint(-10, 40) # store its value and the converted value pair_arr[:, i] = x, celsius2fahrenheit(x)
Arrays In Python: The Complete Guide With Practical Examples
Arrays are one of the fundamental data structures in programming, and Python offers several ways to work with them. When I first started working with Python more than a decade ago, understanding arrays was a game-changer for handling collections of data efficiently.
Store Multiple Values in Python Arrays: The Ultimate Guide
Dec 29, 2023 · Python arrays are a powerful data structure that allows you to store multiple values of the same type in a single variable. In this comprehensive guide, we will explore what Python arrays are, how to use them effectively, and the …
Mastering Python Arrays: Efficiently Store and Manage Similar Data
This article explored Python Arrays, which are data structures used to store similar data types in contiguous memory locations. We discussed the characteristics of Python Arrays, their advantages over Lists, and the differences between them.
Python Array of Numeric Values - Programiz
In this tutorial, we will focus on a module named array. The array module allows us to store a collection of numeric values. Note: When people say arrays in Python, more often than not, they are talking about Python lists. If that's the case, visit the Python list tutorial.
Declaring an Array in Python - GeeksforGeeks
Sep 26, 2023 · Declare Array Using the Array Module in Python. In Python, array module is available to use arrays that behave exactly same as in other languages like C, C++, and Java. It defines an object type which can compactly represent an array of primary values such as integers, characters, and floating point numbers. Syntax to Declare an array
Everything You Need to Know about Python Arrays - Simplilearn
Jun 14, 2024 · Arrays, a fundamental data structure, provide a streamlined way to store multiple values of the same type in a single variable. This tutorial is designed to introduce you to the concept of arrays in Python and showcase how they can be created, accessed, modified, and utilized to optimize your code.