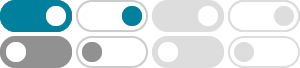
Sort an array in Java - Stack Overflow
Aug 1, 2017 · just FYI, you can now use Java 8 new API for sorting any type of array using parallelSort. parallelSort uses Fork/Join framework introduced in Java 7 to assign the sorting tasks to multiple threads available in the thread pool. the two methods that can be used to sort int array, parallelSort(int[] a) parallelSort(int[] a,int fromIndex,int toIndex)
How to sort an array of objects in Java? - Stack Overflow
One way is to use a standard sorting algorithm. Let's say you have an array of books and you want to sort them on their height, which is stored as an int and accessible through the method getHeight(). Here's how you could sort the books in your array. (If you don't want to change the original array, simply make a copy and sort that.)
java Arrays.sort 2d array - Stack Overflow
Jan 5, 2012 · Sorting a 2d array in Java. 0. Sort 2d arrays Using Arrays.sort in java. Hot Network Questions ICMJE ...
Sort array from smallest to largest using java - Stack Overflow
Feb 15, 2013 · I'm supposed to create an array and sort the numbers from smallest to largest. Here is what I have so far: public class bubbleSort { public static void sort (int [] arrayName){ int temp; ...
Java Array Sort descending? - Stack Overflow
Nov 7, 2009 · Sorting an array in ascending order in java. 0. Int array sorting itself. Hot Network Questions Feeling ...
java - How to sort a List/ArrayList? - Stack Overflow
Apr 27, 2013 · Collections.sort allows you to pass an instance of a Comparator which defines the sorting logic. So instead of sorting the list in natural order and then reversing it, one can simply pass Collections.reverseOrder() to sort in order to sort the list in reverse order: // import java.util.Collections; Collections.sort(testList, Collections ...
Using a For Loop to Manually Sort an Array - Java
Jan 12, 2016 · You have to initialize the value for j as i+1, this sorting algorithm is called bubble sort, that works by repeatedly swapping the adjacent elements if they are in wrong order. The below method is always runs O(n^2) time even if the array is sorted.
java - Sorting an int array from highest to lowest - Stack Overflow
Nov 27, 2013 · If you have an int[] array, you can sort it using Arrays.sort and then reverse it :. int [] tab2 = new int[]{1,5,0,-2}; Arrays.sort(tab2); ArrayUtils.reverse(tab2 ...
custom sorting a java array - Stack Overflow
May 6, 2012 · You're better off with an Integer array than an int array if you're using a generic Comparator<Integer>. You have to use Arrays.sort instead Collections.sort for sorting an array. You have to make the distances variable final if it's referenced in an anonymous inner class.
java - Sorting two arrays simultaneously - Stack Overflow
Apr 28, 2014 · Java might solve this when Project Valhalla is fully delivered, but until then, you need to use a workaround. Solution without object overhead. Make a third array. Fill it with indices from 0 to size-1. Sort this array with Comparator function peeking into the two arrays according to your sort criteria.