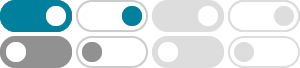
Singleton Design Pattern in Java: 6 Implementations with Code Examples …
Feb 6, 2023 · In Java and other object-oriented languages, that "single thing" is the instance of a class. In short, Singletons are classes that are only allowed to have one instance (or object) during the program execution. Let's use a diagram to illustrate a Singleton class: Figure 1: A UML diagram for the Singleton Design Pattern
Singleton Method Design Pattern - GeeksforGeeks
Jan 3, 2025 · The Singleton pattern or pattern singleton incorporates a private constructor, which serves as a barricade against external attempts to create instances of the Singleton class. This ensures that the class has control over its instantiation process.
Java Singleton Design Pattern Practices with Examples
Jan 3, 2025 · In object-oriented programming, a Java singleton class is a class that can have only one object (an instance of the class) at a time. After the first time, if we try to instantiate the Java Singleton classes, the new variable also points to the first instance created.
The Singleton Design Pattern: A Comprehensive Guide
Mar 30, 2025 · UML Diagram. Before diving into the code, let’s visualize the Singleton pattern with a UML class diagram:
How do I mark a class as a singleton in UML? - Stack Overflow
Dec 5, 2011 · To specify that a class is a singleton, you can write a constraint in between braces: { number of instances = 1 }. This constraint should be put in a constraints compartment in the class rectangle. The UML 2.5 specification, §7.6.4 defines the notation for constraints in general and §9.2.4 specifies how to show the constraints of a classifier:
5 Ways to implement Singleton Design Pattern in Java? Examples
May 5, 2020 · Here is the UML diagram of Singleton design pattern in Java and then we'll see how you can implement Singleton pattern in Java: 1. Lazy initialization, non-thread-safe: This is the classical version, but it's not thread-safe. If more than one thread attempts to access instance at the same time, more than one instance may be created.
Java Singleton Design Pattern Best Practices with Examples
Nov 5, 2022 · Singleton pattern restricts the instantiation of a class and ensures that only one instance of the class exists in the Java Virtual Machine. The singleton class must provide a global access point to get the instance of the class. Singleton pattern is used for logging, drivers objects, caching, and thread pool.
Singleton Design Pattern | by Harsh Khandelwal | System Design …
Sep 18, 2024 · Here’s a simple diagram illustrating these rules: -static instance: Singleton. -Singleton() +static getInstance(): Singleton. +someMethod() The -static instance: Singleton represents the...
Design Patterns In Java: Singleton, Factory And Builder
Apr 1, 2025 · Singleton pattern is a design pattern in which only one instance of a class is present in the Java virtual machine. A singleton class (implementing singleton pattern) has to provide a global access point to get the instance of the class. In other words, a singleton pattern restricts the instantiation of a class.
Singleton - refactoring.guru
Singleton is a creational design pattern that lets you ensure that a class has only one instance, while providing a global access point to this instance. The Singleton pattern solves two problems at the same time, violating the Single Responsibility Principle: Ensure that a …
- Some results have been removed