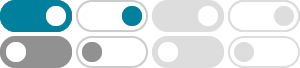
How do I get time of a Python program's execution?
Oct 13, 2009 · I've looked at the timeit module, but it seems it's only for small snippets of code. I want to time the whole program. The simplest way in Python: This assumes that your program takes at least a tenth of second to run. Prints: on Windows, do the same thing, but use time.clock () instead of time.time (). You will get slightly better accuracy.
How do you calculate program run time in python? [duplicate]
For example (if myOwnFunc was locally defined and what you wanted to time): print timeit.timeit('myOwnFunc()', setup='from __main__ import myOwnFunc', number=1). Without the setup parameter, it will complain that it could not find myOwnFunc.
How to check the execution time of Python script - GeeksforGeeks
Apr 26, 2023 · In this article, we will discuss how to check the execution time of a Python script. There are many Python modules like time, timeit and datetime module in Python which can store the time at which a particular section of the program is being executed.
Python RuntimeError (Examples & Use Cases) | Initial Commit
Jan 6, 2023 · Let's take a look at some examples of common run-time errors and how to fix them. A ZeroDivisionError is thrown when you try to divide or modulo by zero in your code, for example: File "<stdin>", line 3, in <module> .
Examples of Runtime Errors in Python - Online Tutorials Library
Oct 31, 2022 · In this article, we will look at some examples of runtime errors in Python Programming Language. A runtime error in a program is one that occurs after the program has been successfully compiled. The Python interpreter will run a program if it is syntactically correct (free of syntax errors).
how to measure running time of algorithms in python
Oct 1, 2016 · To determine the time spent by a real Python program, use the stdlib profiling utilities. This will tell you where in an example program your code is spending its time. Using a decorator for measuring execution time for functions can be handy.
Measure Python Program Execution Time | by Doug Creates
Mar 19, 2024 · Measuring the execution time of a Python program involves tracking the start and end times, then calculating the difference, providing insights into performance and efficiency. This is a recipe...
Top 4 Ways to Accurately Measure Program Run Time in Python
Nov 6, 2024 · If you’re wondering how to measure program run time in Python, here are four effective methods that you can employ. Method 1: Using datetime Module. One straightforward way to measure the execution time of a segment of your Python code is by utilizing the datetime module. Here’s how you can achieve that: ## Start time start_time = datetime.now()
Python Programming Examples | Python Programs - Sanfoundry
Every example program includes the problem description, problem solution, source code, program explanation, and run-time test cases. All Python examples have been compiled and tested on Windows and Linux systems. Python Program to Find the Sum of the Series 1/1!+1/2!+1/3!+…1/N! More Python Programming Examples on Graph.
93+ Python Programming Examples - codingem.com
In this article, you will find a comprehensive list of Python code examples that cover most of the basics. This list of 100 useful Python examples is intended to support someone who is: Preparing for a coding interview. Preparing for an examination. Exploring what programming is. Without further ado, let’s get coding! 1.