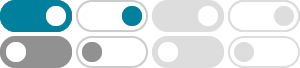
How to reverse a String in Python - GeeksforGeeks
Nov 21, 2024 · Python provides a built-in function called reversed (), which can be used to reverse the characters in a string. This approach is very useful if we prefer to use built-in methods. Explanation: reversed (s) returns an iterator of the characters in s in reverse order. ”.join (reversed (s)) then joins these characters into a new string.
How do I reverse a string in Python? - Stack Overflow
May 31, 2009 · You can do this with pure syntax and literals using a while loop: def reverse_a_string_slowly(a_string): new_string = '' index = len(a_string) while index: index -= 1 # index = index - 1 new_string += a_string[index] # new_string = new_string + …
How to Reverse a String in Python - W3Schools
There is no built-in function to reverse a String in Python. The fastest (and easiest?) way is to use a slice that steps backwards, -1. Reverse the string "Hello World": We have a string, "Hello World", which we want to reverse: Create a slice that starts at …
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing their pros, cons, and performance characteristics.
How to reverse a String in Python (5 Methods)
Jan 24, 2024 · Slicing is a concise and Pythonic way to reverse a string. The [::-1] slice notation indicates a step of -1, effectively reversing the string. Method 2: Using reversed() Function
Reverse a String in Python: Easy Guide - PyTutorial
Feb 7, 2025 · Reversing a string is a common task in Python programming. It can be done in multiple ways. This guide will show you how to reverse a string using slicing, loops, and built-in functions.
Python Reverse String: 7 Effective Ways with Examples
Feb 18, 2025 · One of the most Pythonic ways to reverse a string is by combining the reversed() function with the str.join() method. When you pass a string to reversed(), it doesn’t immediately return a reversed string. Instead, it provides a special reversed iterator object.
Reversing Strings in Python: A Comprehensive Guide
Feb 19, 2025 · Reversing a string in Python can be achieved through multiple methods, each with its own advantages and use cases. Slicing offers a simple and efficient way for basic reversals, while the reversed() function provides more flexibility when working with iterators.
How to Reverse a String in Python
Jul 7, 2022 · Single Quote and Double Quote are used for declaring the single-line string. And we can use an appropriate string declaration with single and double quotes when we have to use an escape sequence or an inside declaration of some text with quotes. Triple Quotes are mostly used for declaring the multiline strings.
Reversing a String in Python: A Comprehensive Guide
Jan 23, 2025 · Python provides a built-in reversed() function that can be used to reverse an iterable, including a string. However, the reversed() function returns an iterator object, so you need to convert it to a string. Here's how you can do it: original_string = "Hello, World!"