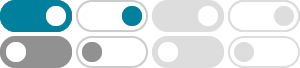
Reverse an array in Java - GeeksforGeeks
Nov 25, 2024 · The simplest way to reverse an array in Java is by using a loop to swap elements or by using the helper methods from the Collections and Arrays classes. Example: Let’s take …
5 Best Ways to Reverse an Array in Java - Codingface
May 20, 2022 · We can reverse the order of an Array in Java by following methods: 1. Reverse an Array by using a simple for loop through iteration. 2. Reverse an Array by using the in-place …
Looping through the elements in an array backwards
Arrays in Java are indexed from 0 to length - 1, not 1 to length, therefore you should be assign your variable accordingly and use the correct comparison operator. Your loop should look like …
Reverse array with for loop in Java - Stack Overflow
Sep 25, 2020 · You don't need a nested loop - you need only one loop to go over the array and assign each element to the corresponding "reversed" array: for (int i = arr.length - 1; i >= 0; i--) …
Can one do a for each loop in java in reverse order?
You can iterate through an array in reverse order using For-Each loop like below: public class Main { public static void main(String[] args) { int arr[] = {2,3,1,4,7,6}; int i = arr.length-1; for(int …
Java – Iterate Array in Reverse Order - GeeksforGeeks
Dec 9, 2024 · We have multiple ways to iterate an array in reverse order. Example 1: The most simplest way to iterate over an array in reverse order is by using a for loop. Example 2: The …
How to Invert an Array in Java - Baeldung
Jan 8, 2024 · The first way we might think about to invert an array is by using a for loop: void invertUsingFor(Object[] array) { for (int i = 0; i < array.length / 2; i++) { Object temp = array[i]; …
Java Program - Reverse an Array - Tutorial Kart
Java Reverse Array - To reverse Array in Java, use for loop to traverse through the array and reverse the array, or use ArrayUtils.reverse() method of Apache's commons.lang package. In …
Array Reverse – Complete Tutorial - GeeksforGeeks
Sep 25, 2024 · Given an array arr[], the task is to reverse the array. Reversing an array means rearranging the elements such that the first element becomes the last, the second element …
Reverse An Array In Java - 3 Methods With Examples - Software …
Apr 1, 2025 · Q #1) How do you Reverse an Array in Java? Answer: There are three methods to reverse an array in Java. Using a for loop to traverse the array and copy the elements in …