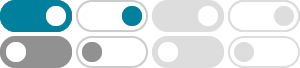
JavaScript Program to Reverse Digits of a Number
Aug 21, 2024 · In this approach, using string iteration,we convert number to string, iterate backward using a loop, and construct reversed string by appending digits. Convert back to a number. let numStr = num.toString(); let reversedStr = ''; for (let i = numStr.length - 1; i >= 0; i--) { reversedStr += numStr[i]; return parseInt(reversedStr);
JavaScript: How to reverse a number? - Stack Overflow
Jun 27, 2016 · Using JavaScript reverse() and Math.sign() you can reverse a number both positive and negative numbers. var enteredNum = prompt("Enter integer"); function reverseInteger(enteredNum) { const reveredNumber = enteredNum.toString().split('').reverse().join(''); return parseInt(reveredNumber)*Math.sign(enteredNum); } alert(reverseInteger(enteredNum));
How do I reverse a number in javascript using a for loop?
Oct 3, 2022 · For a project, I am supposed to write a function in javascript that takes a value and converts it to binary (from decimal) and I need a step to be able to reverse the digits of a number (for the remainders) and I am unsure of how to do it, within the restrictions of using a loop.
Reverse Number in JavaScript Using For Loop: A Guide
Sep 15, 2023 · This comprehensive guide aims to provide a clear understanding of utilizing ‘for loops’ in JavaScript, but with a twist—we will focus on how to run them in reverse. If you’re eager to explore this less commonly used but incredibly useful technique, then this article is …
javascript - How can i reverse numbers with loop - Stack Overflow
Jan 12, 2017 · reverse = reverse * 10 + remainder; n = n / 10; The only missing term is rounding of the number to nearest integer. Here is updated code. remainder = n%10; reverse = reverse * 10 + remainder; n = Math.floor(n / 10); Try console.log (352/10); and console.log (Math.floor (352/10); You will understand it. n = parseInt(n / 10); n = n / 10;
How to reverse a number in JavaScript - CodeVsColor
Apr 8, 2023 · You will learn how to use a while loop, how to use the modulo operation, and how to find the floor value of a number in JavaScript with these examples. We will also write it with HTML/CSS to calculate the reverse of a number by getting the number as input from the user.
Reverse a Number in Javascript - Scaler
May 29, 2024 · There are many methods that can be used to reverse a number in JavaScript: Using the algorithm to reverse a number; By Converting the number to a string and using the below methods: split() reverse() join() Solving with an Arrow Function; Solving with a …
How to Reverse a Number in JavaScript: A Comprehensive …
Aug 30, 2024 · Use Modulo. The modulo operator (%) extracts the last digit, which we can prepend using math operators. Recursive Approach. Functions that call themselves avoid loops by repeating logic in a declarative format. Iterative Approach. Classic loops like for, while, do-while enable iteration without function calls.
How to reverse a number in JavaScript - freeCodeCamp.org
Feb 3, 2019 · num.reverse() reverses the order of the items in the array. num.join('') reassembles the reversed characters into a String. parseFloat(num) converts num into a float from a String. Note: parseFloat runs in the end (even though it is on the first line of the function) on the reversed number and removes any leading zeroes.
JavaScript Code to Reverse a Number – TecAdmin
Jul 12, 2023 · We can reverse a number in JavaScript by following these steps: Convert the number into a string using the `toString()` method. Split the string into an array of characters using the `split(”)` method.
- Some results have been removed