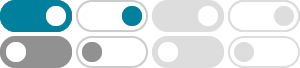
Python Program to Reverse a Number - GeeksforGeeks
Feb 26, 2025 · In this example, in below code Python function `reverse_number` recursively reverses a given number by extracting the last digit and placing it at the appropriate position. The recursion continues until the original number is fully reversed. The example demonstrates reversing the number 987654.
How to Reverse a Number in Python? - Python Guides
Mar 20, 2025 · Learn how to reverse a number in Python using loops, recursion, and string manipulation. Explore different methods to efficiently reverse digits in Python.
Python: Reverse a Number (3 Easy Ways) - datagy
Oct 23, 2021 · Python makes it easy to reverse a number by using a while loop. We can use a Python while loop with the help of both floor division and the modulus % operator. Let’s take a look at an example to see how this works and then dive into why this works: digit = number % 10 . reversed_number = reversed_number * 10 + digit.
Reverse a Number in Python using Function - Know Program
We will develop a program to reverse a number in python using function. To reverse a number we need to extract the last digit of the number and add that number into a temporary variable with some calculation, then remove the last digit of the number. Do …
Python Program to Reverse a Number
Write a function to reverse a given number. For example, for input 12345, the output should be 54321. Did you find this article helpful? In this example, you will learn to reverse a number.
Python Program For Reverse Of A Number (3 Methods With Code) - Python …
How do you reverse a number in Python? To reverse a number in Python, you can use the following steps: Convert the number to a string. Use string slicing with a step of -1 to reverse the string. Convert the reversed string back to an integer. Here’s an example code snippet that demonstrates this approach. Python Program For Reverse Of A Number
How to reverse an int in python? - Stack Overflow
Jul 25, 2014 · Use n //= 10 instead of n /= 10. This will result small number and will not return a ideal reversed number, a infinite number inf. Something like this? You are approaching this in quite an odd way. You already have a reversing function, so why not make line just build the line the normal way around? return "{0} {1} {2}".format(bottles, .
Reverse a Number in Python using inbuilt Function - Know …
We will develop a program to reverse a number in python using inbuilt function. To reverse a number we need to extract the last digit of the number and add that number into a temporary variable with some calculation, then remove the last digit of the number. Do these processes until the number becomes zero.
Reverse a string or number in python - Devsheet
Jan 4, 2023 · To reverse a string in Python: Create a string my_str = "hello". Use string [::-1] to reverse the string my_str [::-1]. The above code example shows the fastest way to reverse a Python String or a number. We just pass the [::-1] after the variable name to reverse a …
How to Reverse a Number in Python - updategadh.com
Jan 28, 2025 · Method 2: Reverse a Number Using Recursion. Recursion is another effective way to reverse a number. Let’s look at the logic and implementation. Algorithm. Input: An integer number. Create a global variable reversed_number = 0. If number > 0: Extract the remainder of the number when divided by 10. Multiply reversed_number by 10 and add the ...